TypeError: argument of type 'numpy.dtype[object_]' is not iterable
时间: 2023-07-02 13:05:08 浏览: 238
这个错误通常是因为你试图迭代一个 Numpy 数组的 dtype 对象,而 dtype 对象是不可迭代的。请检查你的代码,确保你正在尝试迭代数组本身,而不是 dtype 对象。以下是一个例子,将 Numpy 数组转换为列表:
```python
import numpy as np
# 创建一个 Numpy 数组
arr = np.array([1, 2, 3])
# 错误的写法,尝试迭代 dtype 对象
for item in arr.dtype:
print(item)
# 正确的写法,将数组转换为列表后迭代
for item in arr.tolist():
print(item)
```
在上面的例子中,我们首先创建了一个 Numpy 数组 `arr`,然后尝试迭代 `arr.dtype`,导致了 TypeError。我们通过将数组转换为列表来解决这个问题,并通过 `tolist()` 方法将数组转换为列表,然后迭代列表中的元素。
相关问题
TypeError: argument of type 'numpy.int64' is not iterable
这个错误通常会出现在使用NumPy中的某些函数时,因为该函数需要一个可迭代的对象作为输入参数,但输入了一个整数或其他不可迭代的对象。
可能的解决方案:
1. 检查代码中使用的所有NumPy函数,并确保传递给它们的参数是可迭代的对象。
2. 如果输入参数是整数,可以将其转换为列表或数组,以便可以进行迭代。例如,可以使用`[number]`将整数转换为包含该整数的列表。
3. 如果您不确定哪个函数导致了错误,请逐步调试代码并打印变量以查找问题。
TypeError: argument of type 'numpy.float64' is not iterable
This error occurs when you try to iterate over a numpy float64 object, which is not iterable.
For example, the following code will raise this error:
```python
import numpy as np
x = np.float64(3.14)
for i in x:
print(i)
```
To fix this error, you need to convert the numpy float64 object to an iterable object such as a list or an array.
For example:
```python
import numpy as np
x = np.float64(3.14)
lst = [x] # Convert x to a list
for i in lst:
print(i)
```
Alternatively, you may want to check your code and make sure that you are not accidentally trying to iterate over a numpy float64 object when you meant to iterate over a list or an array.
阅读全文
相关推荐
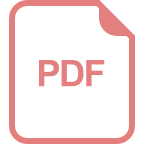
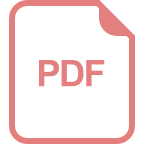














