怎么用c语言输入英语单词
时间: 2023-04-02 18:04:16 浏览: 311
你可以使用 scanf 函数来输入英语单词,例如:
```c
char word[20];
scanf("%s", word);
```
这将允许用户在程序运行时输入一个英语单词,并将其存储在名为 word 的字符数组中。请注意,这种方法只适用于单词,如果需要输入一个包含空格的短语,则需要使用其他方法。
相关问题
怎么用c语言将英语单词的单数变复数形式
可以使用字符串操作函数来实现将英语单词的单数变成复数形式。具体步骤如下:
1. 判断单词的结尾字母,如果是s、x、z、ch、sh结尾,则在单词末尾加上es,如box变成boxes。
2. 如果单词结尾是y,则将y替换成ies,如baby变成babies。
3. 如果单词结尾是f或fe,则将f或fe替换成ves,如knife变成knives。
4. 如果单词结尾是o,则在单词末尾加上es,如potato变成potatoes。
5. 其他情况,在单词末尾加上s,如book变成books。
下面是一个示例代码:
#include <stdio.h>
#include <string.h>
void pluralize(char *word) {
int len = strlen(word);
char last = word[len-1];
if (last == 's' || last == 'x' || last == 'z' ||
(len >= 2 && word[len-2] == 'c' && last == 'h') ||
(len >= 2 && word[len-2] == 's' && last == 'h')) {
strcat(word, "es");
} else if (last == 'y') {
word[len-1] = 'i';
strcat(word, "es");
} else if (len >= 2 && word[len-2] == 'f' && last == 'e') {
word[len-2] = 'v';
word[len-1] = 'e';
strcat(word, "s");
} else if (last == 'o') {
strcat(word, "es");
} else {
strcat(word, "s");
}
}
int main() {
char word[100];
printf("请输入一个英语单词:");
scanf("%s", word);
pluralize(word);
printf("复数形式为:%s\n", word);
return ;
}
C语言输入句子提取单词
要提取单词,需要先了解单词的定义。在英语中,单词是由字母组成的、表示一个特定含义的字符串。因此,提取单词就是要从输入的句子中找出由字母组成的字符串。
以下是一个示例代码,可以输入一个英文句子,然后提取出其中的单词并打印出来:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int main() {
char sentence[1000]; // 存储输入的句子
char word[100]; // 存储提取出来的单词
int sentence_len, i, j, word_start;
printf("请输入一个英文句子:\n");
fgets(sentence, 1000, stdin); // 从标准输入读入句子
sentence_len = strlen(sentence);
i = 0;
while (i < sentence_len) {
// 找到单词的起始位置
while (i < sentence_len && !isalpha(sentence[i])) {
i++;
}
if (i == sentence_len) {
break;
}
// 找到单词的结束位置
j = i;
while (j < sentence_len && isalpha(sentence[j])) {
j++;
}
// 将单词复制到 word 数组中并打印出来
word_start = i;
strncpy(word, sentence + word_start, j - word_start);
word[j - word_start] = '\0';
printf("%s\n", word);
i = j;
}
return 0;
}
```
该代码的大致思路是:
1. 先输入一个英文句子,存储在字符数组 `sentence` 中。
2. 定义另一个字符数组 `word`,用来存储提取出来的单词。
3. 定义变量 `sentence_len` 表示句子的长度,`i` 和 `j` 分别表示遍历句子和查找单词的位置,`word_start` 表示单词在句子中的起始位置。
4. 在一个循环中,不断查找单词的起始位置和结束位置,然后将单词复制到 `word` 数组中并打印出来。
5. 循环结束后程序退出。
在代码中,使用了 C 语言中的一些库函数:
- `fgets` 用来从标准输入读入句子。
- `isalpha` 用来判断一个字符是否为字母。
- `strlen` 用来获取一个字符串的长度。
- `strncpy` 用来将一个字符串复制到另一个字符串中。
相关推荐
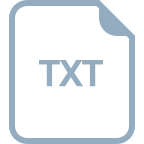
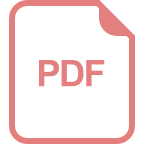
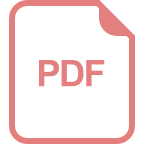












