c++ 实现定时监控进程,若未打开则重启带UI界面的进程
时间: 2023-08-18 20:09:57 浏览: 174
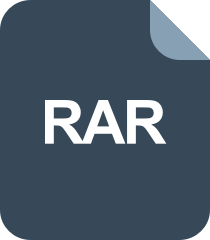
用C++写的进程管理

可以使用Windows API来实现定时监控进程,以下是一个简单的实现方法:
```c++
#include <windows.h>
#include <tlhelp32.h>
#include <string>
using namespace std;
// 打开进程,返回进程句柄
HANDLE OpenProcessByName(const string& processName)
{
PROCESSENTRY32 pe32;
HANDLE hSnapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (hSnapshot == INVALID_HANDLE_VALUE) {
return NULL;
}
pe32.dwSize = sizeof(PROCESSENTRY32);
if (!Process32First(hSnapshot, &pe32)) {
CloseHandle(hSnapshot);
return NULL;
}
do {
if (processName == pe32.szExeFile) {
CloseHandle(hSnapshot);
return OpenProcess(PROCESS_ALL_ACCESS, FALSE, pe32.th32ProcessID);
}
} while (Process32Next(hSnapshot, &pe32));
CloseHandle(hSnapshot);
return NULL;
}
// 判断进程是否存在
bool IsProcessExist(const string& processName)
{
HANDLE hProcess = OpenProcessByName(processName);
if (hProcess != NULL) {
CloseHandle(hProcess);
return true;
}
return false;
}
// 启动进程
void StartProcess(const string& processPath)
{
STARTUPINFO si = { 0 };
PROCESS_INFORMATION pi = { 0 };
CreateProcess(processPath.c_str(), NULL, NULL, NULL, FALSE, 0, NULL, NULL, &si, &pi);
}
int main()
{
string processName = "process.exe";
string processPath = "D:\\process.exe";
// 定时检查进程是否存在
while (true) {
Sleep(5000); // 每5秒检查一次
if (!IsProcessExist(processName)) {
StartProcess(processPath);
}
}
return 0;
}
```
上面的代码中,`OpenProcessByName`函数用于打开指定进程,`IsProcessExist`函数用于判断进程是否存在,`StartProcess`函数用于启动指定的进程。通过在一个循环中定时执行这些函数,就可以实现监控进程并重启带UI界面的进程的功能。
阅读全文
相关推荐

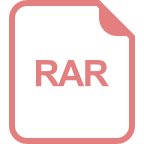
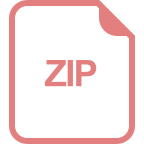
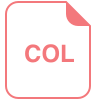
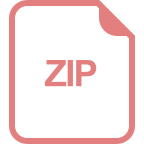
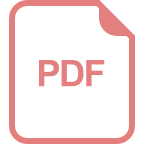
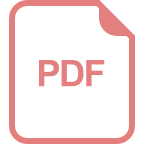
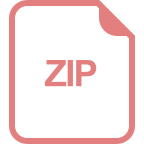
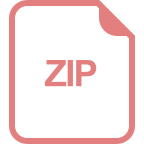
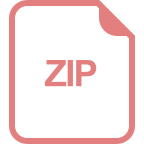
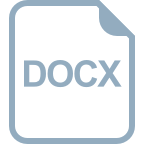
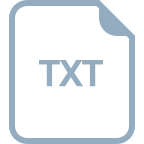