c#怎么把像素值转换为矩阵
时间: 2023-06-23 19:08:49 浏览: 91
在C#中,可以使用`Bitmap`类来获取图像的像素矩阵。`Bitmap`类提供了`GetPixel()`和`SetPixel()`方法来获取和设置指定坐标的像素值。
可以使用以下代码将位图转换为像素值矩阵:
```csharp
Bitmap bmp = new Bitmap("image.bmp");
int width = bmp.Width;
int height = bmp.Height;
Color pixelColor;
int[,] pixels = new int[width, height];
for (int i = 0; i < width; i++)
{
for (int j = 0; j < height; j++)
{
pixelColor = bmp.GetPixel(i, j);
pixels[i, j] = pixelColor.ToArgb();
}
}
```
在上述代码中,首先加载图像文件并获取图像的宽度和高度。然后使用嵌套循环遍历所有像素,并使用`GetPixel()`方法获取每个像素的颜色值。最后,将颜色值转换为整数并存储在矩阵中。
请注意,此方法可能会导致性能问题,因为对于大型图像,遍历所有像素可能会非常耗时。
相关问题
c#怎么把灰度化以后的图像转换为矩阵
可以使用C#中的Bitmap类来读取灰度化后的图像。然后,可以使用GetPixel方法从图像中获取每个像素的颜色值,将其转换为灰度值,并将其存储在一个数组或矩阵中。
以下是一个示例代码,将灰度化后的图像转换为矩阵:
```csharp
Bitmap image = new Bitmap("gray_image.jpg");
int width = image.Width;
int height = image.Height;
int[,] matrix = new int[width, height];
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
Color pixelColor = image.GetPixel(x, y);
// 获取灰度值
int grayValue = (int)(pixelColor.R * 0.3 + pixelColor.G * 0.59 + pixelColor.B * 0.11);
// 存储灰度值到矩阵中
matrix[x, y] = grayValue;
}
}
```
在上面的代码中,我们使用了GetPixel方法从图像中获取每个像素的颜色值,并将其转换为灰度值。然后,我们将灰度值存储在一个二维数组或矩阵中。最终,我们可以使用这个矩阵来进行进一步的图像处理或分析。
C# 图像数据转成矩阵
在C#中,可以使用Bitmap类读取图像数据,并将其转换为矩阵。
以下是一个示例代码:
```csharp
// 读取图像文件
Bitmap bitmap = new Bitmap("image.jpg");
// 获取图像的宽度和高度
int width = bitmap.Width;
int height = bitmap.Height;
// 创建一个与图像大小相同的二维数组
int[,] matrix = new int[width, height];
// 将每个像素的 RGB 值转换为灰度值,并存储到矩阵中
for (int x = 0; x < width; x++)
{
for (int y = 0; y < height; y++)
{
Color pixel = bitmap.GetPixel(x, y);
int gray = (int)(0.299 * pixel.R + 0.587 * pixel.G + 0.114 * pixel.B);
matrix[x, y] = gray;
}
}
```
在上面的示例中,我们使用GetPixel方法从Bitmap对象中获取每个像素的RGB值,并使用以下公式将它们转换为灰度值:
灰度值 = 0.299 * R + 0.587 * G + 0.114 * B
然后,我们将每个像素的灰度值存储到矩阵中。
相关推荐
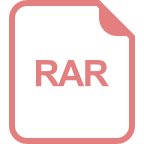
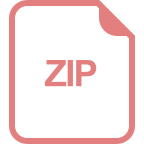
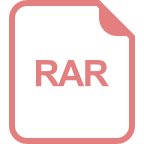






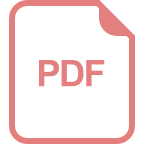
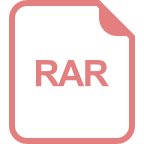
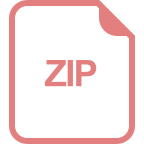
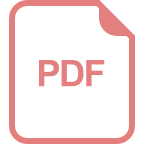
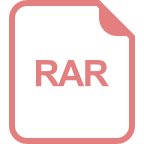
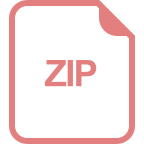
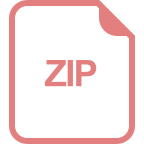