@Override public boolean findUserInfoByIdAndName(@RequestParam Integer name, @RequestParam String age) throws Exception { if (name == null) { throw new Exception("用户名不能为空"); } if (age == null) { throw new Exception("年龄不能为空"); } String ageStr = "18"; if (age < 120) { return true; } throw new Exception("年龄必须大于18且小于120"); UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name,age); if (userInfo == null) { throw new Exception("未找到符合条件的用户信息"); }
时间: 2024-02-15 16:28:47 浏览: 6
这段代码是一个Java方法,它的作用是根据用户名和年龄查询用户信息。方法的参数包括一个整型的name和一个字符串类型的age,返回一个布尔类型的值。
在方法中,首先会进行参数的非空校验,如果参数为空,则抛出异常。然后会对年龄进行判断,如果年龄小于18或大于120,则抛出异常。
接下来会调用一个名为findUserInfoByIdAndName的方法,该方法会根据传入的用户名和年龄进行查询用户信息的操作。如果查询结果为null,则表示未找到符合条件的用户信息,会抛出异常。
最后,如果整个方法执行成功,会返回true。
相关问题
@Override public boolean findUserInfoByIdAndName(@RequestParam Integer name, @RequestParam String age) throws Exception { if (name == null) { throw new Exception("用户名不能为空"); } if (age == null) { throw new Exception("年龄不能为空"); } String ageStr = "18"; if (age < 120) { return true; } throw new Exception("年龄必须大于18且小于120"); UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name,age); if (userInfo == null) { throw new Exception("未找到符合条件的用户信息"); }优化下面的代码
以下是对该段代码的一些优化建议:
1. 参数校验可以使用注解@NotBlank和@NotNull来简化代码。
2. 年龄的判断可以改为使用Integer类型进行比较。
3. 异常信息可以封装成常量或枚举类型,方便维护和修改。
4. 代码中有一行无法执行到的语句,应该进行删除。
优化后的代码如下所示:
```
@Override
public boolean findUserInfoByIdAndName(@NotBlank(message = "用户名不能为空") Integer name,
@NotNull(message = "年龄不能为空") Integer age) throws Exception {
final Integer MIN_AGE = 18;
final Integer MAX_AGE = 120;
if (age < MIN_AGE || age > MAX_AGE) {
throw new Exception("年龄必须大于18且小于120");
}
UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name, age);
if (userInfo == null) {
throw new Exception("未找到符合条件的用户信息");
}
return true;
}
```
优化代码中的问题,简洁的代码 @Override public boolean findUserInfoByIdAndName(int age, String name) throws Exception { if (StringUtils.isEmpty(name)) { throw new Exception("用户名不能为空"); } if (age == null) { throw new Exception("年龄不能为空"); } final Integer MIN_AGE = 18; final Integer MAX_AGE = 120; if (age < MIN_AGE || age > MAX_AGE) { throw new Exception("年龄必须大于18且小于120"); } UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name, age); if (userInfo == null) { throw new Exception("未找到符合条件的用户信息"); } return true; } }
可以优化的地方如下:
1. 可以使用Java8的Optional类来避免null的判断,也可以使用Objects.requireNonNull方法来抛出空指针异常。
2. 可以在方法签名中使用基本数据类型,而不是其包装类型。
3. 可以使用自定义异常类来替换Exception,以便更好地描述错误原因。
4. 可以使用静态导入来简化代码。
下面是优化后的代码示例:
```
@Override
public boolean findUserInfoByIdAndName(int age, String name) throws UserNotFoundException, InvalidParameterException, NullPointerException {
Objects.requireNonNull(name, "用户名不能为空");
if (age < 18 || age > 120) {
throw new InvalidParameterException("年龄必须大于18且小于120");
}
UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name, age);
if (userInfo == null) {
throw new UserNotFoundException("未找到符合条件的用户信息");
}
return true;
}
```
注意,这个示例中的UserNotFoundException和InvalidParameterException需要自定义实现。
相关推荐
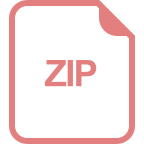
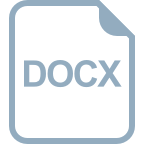
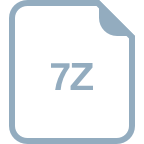













