JAVA实现模板word文档导入的具体代码
时间: 2023-03-13 15:30:35 浏览: 376
答:可以使用Apache POI库来实现JAVA代码的模板word文档导入,具体的代码如下:FileInputStream inputStream = new FileInputStream("document.docx");
XWPFDocument doc = new XWPFDocument(inputStream);
List<XWPFParagraph> paragraphs = doc.getParagraphs();
for (XWPFParagraph paragraph : paragraphs) {
// do something with the paragraph
}
相关问题
写一段java代码实现导入word模板并生成一份word
文档,其中需要替换掉模板中的某些变量。
以下是一段示例代码,其中使用了Apache POI库进行操作:
```
import java.io.*;
import org.apache.poi.xwpf.usermodel.*;
public class WordGenerator {
public static void main(String[] args) throws Exception {
// 读取模板文件
FileInputStream inputStream = new FileInputStream("template.docx");
XWPFDocument document = new XWPFDocument(inputStream);
// 替换变量
for (XWPFParagraph p : document.getParagraphs()) {
List<XWPFRun> runs = p.getRuns();
if (runs != null) {
for (XWPFRun r : runs) {
String text = r.getText(0);
if (text != null && text.contains("{{var1}}")) {
text = text.replace("{{var1}}", "替换后的文本1");
r.setText(text, 0);
}
if (text != null && text.contains("{{var2}}")) {
text = text.replace("{{var2}}", "替换后的文本2");
r.setText(text, 0);
}
}
}
}
// 输出生成的文档
FileOutputStream outputStream = new FileOutputStream("output.docx");
document.write(outputStream);
outputStream.close();
}
}
```
上述代码中,我们首先读取了一个名为“template.docx”的word模板文件。然后,遍历每个段落和文本对象,查找其中包含的特定字符串,并将其替换为我们想要的文本。最后,我们将生成的文档输出到一个名为“output.docx”的文件中。请注意,上述代码仅仅是一份示例代码,实际应用中可能需要根据具体场景进行修改。
java实现用freemarker导出word文档图片循环
使用Java实现使用Freemarker导出Word文档中的图片循环,可以按照以下步骤进行操作:
1. 首先,确保你已经安装了Java开发环境以及Freemarker的jar包。
2. 创建一个Java项目,并在项目中导入Freemarker的相关依赖。
3. 导入需要导出的Word文档文件,并将其中的图片按照你的需求进行命名和分类。
4. 创建一个包含图片路径和其他相关信息的数据结构,例如使用Java的集合类来存储每个图片的路径和文件名。
5. 在Java代码中,使用Freemarker的API来加载并解析Word文档的模板。
6. 在模板文件中,使用Freemarker的语法和标签来定义需要插入图片的位置和循环结构。
7. 在Java代码中,将图片路径和其他相关信息与模板进行结合,生成最终的Word文档。
8. 最后,保存生成的Word文档到指定的位置,并验证导出的效果。
需要注意的是,以上步骤只是一个简单的概括,具体的实现需要根据你的具体需求进行调整和扩展。同时,你还可以使用其他的Java库或工具来辅助实现图片的循环导出,例如Apache POI等。
相关推荐
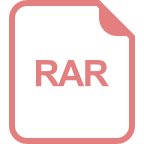












