用python实现互信息和联合熵
时间: 2023-10-28 17:06:14 浏览: 53
好的,我可以帮你解答这个问题。
首先,需要导入 math 和 collections 模块:
```python
import math
import collections
```
然后,我们需要定义一个函数来计算互信息:
```python
def mutual_information(word1, word2, corpus):
word_freq = collections.Counter(corpus)
bigram_freq = collections.Counter(zip(corpus[:-1], corpus[1:]))
total_words = sum(word_freq.values())
p_word1 = word_freq[word1] / total_words
p_word2 = word_freq[word2] / total_words
p_word1_word2 = bigram_freq[(word1, word2)] / (total_words - 1)
return math.log2(p_word1_word2 / (p_word1 * p_word2))
```
其中,参数 word1 和 word2 分别表示要计算互信息的两个词,参数 corpus 是语料库,是一个由词组成的列表。
接下来,我们需要定义一个函数来计算联合熵:
```python
def joint_entropy(word1, word2, corpus):
word_freq = collections.Counter(corpus)
bigram_freq = collections.Counter(zip(corpus[:-1], corpus[1:]))
total_words = sum(word_freq.values())
p_word1_word2 = bigram_freq[(word1, word2)] / (total_words - 1)
return -math.log2(p_word1_word2)
```
同样,参数 word1 和 word2 分别表示要计算联合熵的两个词,参数 corpus 是语料库。
最后,我们可以使用以下代码来测试我们的函数:
```python
corpus = ['this', 'is', 'a', 'test', 'sentence', 'for', 'calculating', 'mutual', 'information', 'and', 'joint', 'entropy']
word1 = 'a'
word2 = 'test'
print(mutual_information(word1, word2, corpus))
print(joint_entropy(word1, word2, corpus))
```
这里的语料库是一个简单的例子,你可以根据你的需求替换为你的语料库。
相关推荐
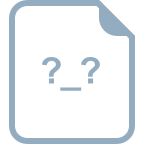










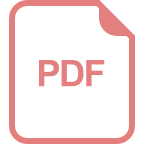
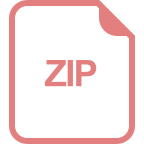