写函数creat(),使其建立单链表来存放学生的信息及输出各学生的信息; (2)写函数delete(),按学号检索学生信息,如果找到,则删除该学生信息,并输出删除过后的所有学生信息,否则输出“不存在!”。 (3)写函数输出链表中的结点。 (4)释放链表所占内存空间。
时间: 2024-03-09 18:50:03 浏览: 97
好的,我会根据你的要求来回答你的问题。以下是我提供的解决方案:
1. 编写 `creat()` 函数来建立单链表并存储学生信息:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
int age;
Student* next;
};
Student* creat() {
Student* head = new Student;
head->next = NULL;
Student* p = head;
int id, age;
string name;
while (true) {
cout << "请输入学生的学号(输入-1结束):";
cin >> id;
if (id == -1) {
break;
}
cout << "请输入学生的姓名:";
cin >> name;
cout << "请输入学生的年龄:";
cin >> age;
Student* s = new Student;
s->id = id;
s->name = name;
s->age = age;
s->next = NULL;
p->next = s;
p = s;
}
return head;
}
```
2. 编写 `delete()` 函数来按学号删除学生信息:
```c++
void delete(Student* head) {
int id;
cout << "请输入要删除的学生学号:";
cin >> id;
Student* p = head->next;
Student* q = head;
while (p != NULL) {
if (p->id == id) {
q->next = p->next;
delete p;
cout << "删除成功!" << endl;
return;
}
q = p;
p = p->next;
}
cout << "不存在!" << endl;
}
```
3. 编写 `print()` 函数来输出链表中的信息:
```c++
void print(Student* head) {
Student* p = head->next;
while (p != NULL) {
cout << "学号:" << p->id << " 姓名:" << p->name << " 年龄:" << p->age << endl;
p = p->next;
}
}
```
4. 编写 `free()` 函数来释放链表所占用的内存空间:
```c++
void free(Student* head) {
Student* p = head->next;
while (p != NULL) {
Student* q = p;
p = p->next;
delete q;
}
delete head;
}
```
以上就是我提供的解决方案,你可以根据自己的需要进行修改和完善。同时,我也提醒你注意代码的规范和注释的添加,这样有助于提高代码的可读性和易维护性。
阅读全文
相关推荐
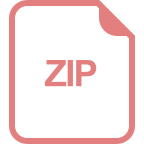



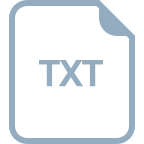
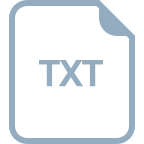
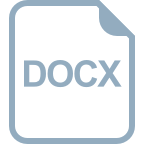
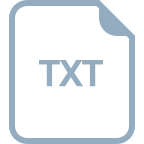







