(1)写函数creat(),使其建立单链表来存放学生的信息及输出各学生的信息; (2)写函数delete(),按学号检索学生信息,如果找到,则删除该学生信息,并输出删除过后的所有学生信息,否则输出“不存在!”。 (3)写函数输出链表中的结点。 (4)释放链表所占内存空间。
时间: 2024-03-09 19:50:55 浏览: 42
好的,我会根据你的要求来回答问题,下面是对你的问题的回答:
1. 首先,我们需要定义一个学生信息的结构体,包括学号、姓名、年龄、性别等字段。然后,我们定义一个单链表结构体,包括头结点和尾结点指针,以及链表长度等字段。接着,我们可以实现一个 creat() 函数,代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char id[10];
char name[20];
int age;
char sex[10];
};
struct Node {
struct Student data;
struct Node *next;
};
struct List {
struct Node *head;
struct Node *tail;
int length;
};
void creat(struct List *list) {
list->head = list->tail = NULL;
list->length = 0;
printf("请输入学生信息,以#结束\n");
while (1) {
struct Node *node = (struct Node *) malloc(sizeof(struct Node));
scanf("%s", node->data.id);
if (strcmp(node->data.id, "#") == 0) {
free(node);
break;
}
scanf("%s%d%s", node->data.name, &node->data.age, node->data.sex);
node->next = NULL;
if (list->head == NULL) {
list->head = list->tail = node;
} else {
list->tail->next = node;
list->tail = node;
}
list->length++;
}
}
```
2. 接下来,我们需要实现一个 delete() 函数,用于删除指定学号的学生信息。如果找到了该学生信息,则在链表中删除该节点,并输出删除过后的所有学生信息。否则,输出“不存在!”。代码如下:
```c
void delete(struct List *list, char *id) {
struct Node *p = list->head;
struct Node *q = NULL;
while (p != NULL) {
if (strcmp(p->data.id, id) == 0) {
if (p == list->head) {
list->head = p->next;
} else if (p == list->tail) {
list->tail = q;
q->next = NULL;
} else {
q->next = p->next;
}
free(p);
list->length--;
printf("删除成功!剩余学生信息如下:\n");
output(list);
return;
}
q = p;
p = p->next;
}
printf("不存在!\n");
}
```
3. 我们还需要实现一个 output() 函数,用于输出链表中的所有结点信息。代码如下:
```c
void output(struct List *list) {
struct Node *p = list->head;
while (p != NULL) {
printf("%s %s %d %s\n", p->data.id, p->data.name, p->data.age, p->data.sex);
p = p->next;
}
}
```
4. 最后,我们需要释放链表所占内存空间。代码如下:
```c
void destroy(struct List *list) {
struct Node *p = list->head;
while (p != NULL) {
struct Node *temp = p;
p = p->next;
free(temp);
}
list->head = list->tail = NULL;
list->length = 0;
}
```
以上就是我对你提出的问题的回答,希望能够帮助到你。
相关推荐
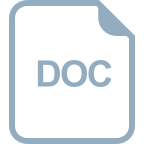
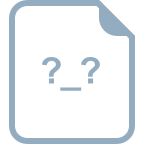














