java label二维数组
时间: 2024-05-28 20:08:15 浏览: 22
在Java中,二维数组是由一维数组组成的数组。我们可以使用标签来标记二维数组,以便在需要的时候更容易地引用它们。Java中的标签是一个带有冒号的标识符,它可以用于任何语句之前。
以下是一个示例代码片段,演示了如何声明和初始化带有标签的二维数组:
```
int[][] arr = new int[3];
outerloop:
for (int i = 0; i < arr.length; i++) {
innerloop:
for (int j = 0; j < arr[i].length; j++) {
if (arr[i][j] == 0) {
System.out.println("Found it at: " + i + "," + j);
break outerloop;
}
}
}
```
在这个例子中,我们使用了两个标签outerloop和innerloop来标记外部和内部循环。然后我们在内部循环中使用条件语句来查找数组中第一个值为零的元素,并使用break语句和标签outerloop来退出外部循环。
相关问题
java二维数组怎样生成arff文件
生成arff文件的主要步骤如下:
1. 导入arff文件格式的包:
```
import weka.core.Instances;
import weka.core.converters.ArffSaver;
```
2. 创建Instances对象:
```
Instances data = new Instances(new BufferedReader(new FileReader("data.arff")));
```
3. 设置特征属性:
```
// 设置属性值
ArrayList<Attribute> attributes = new ArrayList<Attribute>();
Attribute attribute1 = new Attribute("attribute1");
Attribute attribute2 = new Attribute("attribute2");
attributes.add(attribute1);
attributes.add(attribute2);
// 设置label值
ArrayList<String> labels = new ArrayList<String>();
labels.add("label1");
labels.add("label2");
// 设置类别属性
Attribute classAttribute = new Attribute("class", labels);
// 将属性设置到Instances对象中
data.setClass(classAttribute);
data.setAttributes(attributes);
```
4. 生成arff文件:
```
ArffSaver saver = new ArffSaver();
saver.setInstances(data);
saver.setFile(new File("data_new.arff"));
saver.writeBatch();
```
完整代码如下:
```
import weka.core.Attribute;
import weka.core.Instances;
import weka.core.converters.ArffSaver;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.ArrayList;
public class GenerateArff {
public static void main(String[] args) throws Exception {
// 读取数据文件
double[][] dataValues = {{1, 2}, {3, 4}, {5, 6}, {7, 8}};
Instances data = new Instances(new BufferedReader(new FileReader("data.arff")));
// 设置属性值
ArrayList<Attribute> attributes = new ArrayList<Attribute>();
Attribute attribute1 = new Attribute("attribute1");
Attribute attribute2 = new Attribute("attribute2");
attributes.add(attribute1);
attributes.add(attribute2);
// 设置label值
ArrayList<String> labels = new ArrayList<String>();
labels.add("label1");
labels.add("label2");
// 设置类别属性
Attribute classAttribute = new Attribute("class", labels);
// 将属性设置到Instances对象中
data.setClass(classAttribute);
data.setAttributes(attributes);
// 生成arff文件
ArffSaver saver = new ArffSaver();
saver.setInstances(data);
saver.setFile(new File("data_new.arff"));
saver.writeBatch();
}
}
```
运行后,会在当前目录下生成一个名为data_new.arff的文件。
帮我编写一个Java代码,用swing组件制作一个课表界面,总体是一个界面布局,分左右两部分,左边部分为网格布局,两行一列,第一行为图片,第二行是四个标签,四个文本框,右边部分是网格布局,三行一列,第一行是一个标签,第二行是两个标签,两个下拉列表,第三行是一个表格,表格是一维数组,表格内容是二维数组
好的,以下是Java代码,它可以实现你所说的课表界面:
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
public class CourseScheduleUI {
public static void main(String[] args) {
JFrame frame = new JFrame("课表界面");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(800, 600);
frame.setLayout(new GridLayout(1, 2));
// 左侧面板
JPanel leftPanel = new JPanel(new GridLayout(2, 1));
JLabel imageLabel = new JLabel(new ImageIcon("image.jpg"));
leftPanel.add(imageLabel);
JPanel inputPanel = new JPanel(new GridLayout(2, 4));
inputPanel.add(new JLabel("标签1"));
inputPanel.add(new JTextField());
inputPanel.add(new JLabel("标签2"));
inputPanel.add(new JTextField());
inputPanel.add(new JLabel("标签3"));
inputPanel.add(new JTextField());
inputPanel.add(new JLabel("标签4"));
inputPanel.add(new JTextField());
leftPanel.add(inputPanel);
// 右侧面板
JPanel rightPanel = new JPanel(new GridLayout(3, 1));
rightPanel.add(new JLabel("标签5"));
JPanel selectPanel = new JPanel();
selectPanel.add(new JLabel("下拉列表1"));
selectPanel.add(new JComboBox<>(new String[]{"选项1", "选项2", "选项3"}));
selectPanel.add(new JLabel("下拉列表2"));
selectPanel.add(new JComboBox<>(new String[]{"选项1", "选项2", "选项3"}));
rightPanel.add(selectPanel);
JTable table = new JTable(new DefaultTableModel(new Object[][]{{"A", "B", "C"}, {"D", "E", "F"}}, new Object[]{"列1", "列2", "列3"}));
rightPanel.add(table);
frame.add(leftPanel);
frame.add(rightPanel);
frame.setVisible(true);
}
}
```
这个代码使用了`JFrame`、`JPanel`、`JLabel`、`JTextField`、`JComboBox`和`JTable`等Swing组件,实现了你所描述的布局和内容。运行这个代码会弹出一个窗口,其中左侧部分包含一个图片和四个标签、文本框,右侧部分包含一个标签、两个下拉列表和一个表格。你可以根据需要进一步修改和完善这个代码。
相关推荐
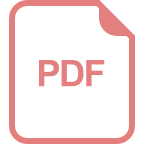
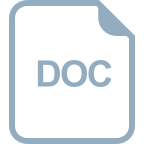
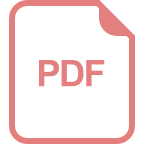











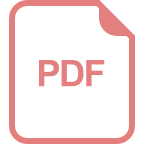
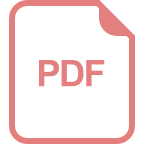