>>> import torch.nn as nn >>> import torch >>> rnn = nn.LSTM(5, 6, 2) >>> input = torch.randn(1, 3, 5) >>> h0 = torch.randn(2, 3, 6) >>> c0 = torch.randn(2, 3, 6) >>> output, (hn, cn) = rnn(input, (h0, c0))
时间: 2023-11-23 12:54:26 浏览: 141
这是一个使用 PyTorch 实现的 LSTM 模型的示例代码。具体来说,这个模型有 5 个输入特征,6 个隐藏单元,2 层 LSTM。输入数据的形状为 (1, 3, 5),其中 1 表示 batch size,3 表示序列长度,5 表示每个时间步的特征数。h0 和 c0 是 LSTM 的初始隐藏状态和细胞状态,形状为 (2, 3, 6),其中 2 表示层数,3 表示 batch size,6 表示隐藏单元数。模型的输出为 output,形状为 (1, 3, 6),表示每个时间步的输出特征,同时还返回了最后一个时间步的隐藏状态 hn 和细胞状态 cn,形状均为 (2, 3, 6)。
相关问题
import torch.nn as nn >>> import torch >>> rnn = nn.LSTM(5, 6, 2) >>> input = torch.randn(1, 3, 5) >>> h0 = torch.randn(2, 3, 6) >>> c0 = torch.randn(2, 3, 6) >>> output, (hn, cn) = rnn(input, (h0, c0)) 打印hn数据后发现其中包含output数据,那么还有一部分数据是什么?
在 LSTM 模型中,每个时间步的输出 output 是根据当前的输入和前一个时间步的隐藏状态计算得到的,而当前时间步的隐藏状态 hn 和细胞状态 cn 则是根据当前的输入和前一个时间步的隐藏状态和细胞状态计算得到的,它们的计算方式如下:
```
i_t = sigmoid(W_{ii} x_t + b_{ii} + W_{hi} h_{t-1} + b_{hi})
f_t = sigmoid(W_{if} x_t + b_{if} + W_{hf} h_{t-1} + b_{hf})
g_t = tanh(W_{ig} x_t + b_{ig} + W_{hg} h_{t-1} + b_{hg})
o_t = sigmoid(W_{io} x_t + b_{io} + W_{ho} h_{t-1} + b_{ho})
c_t = f_t * c_{t-1} + i_t * g_t
h_t = o_t * tanh(c_t)
```
其中,x_t 表示当前时间步的输入,h_{t-1} 和 c_{t-1} 分别表示前一个时间步的隐藏状态和细胞状态,i_t、f_t、g_t 和 o_t 分别表示输入门、遗忘门、记忆门和输出门,W 和 b 分别表示模型的权重和偏置。在上面的代码中,(hn, cn) 是最后一个时间步的隐藏状态和细胞状态,也就是模型经过多个时间步的计算后得到的最终状态,其中包含了所有时间步的信息,包括每个时间步的输入和输出。而 output 只包含每个时间步的输出。
class LSTMNet(torch.nn.Module): def __init__(self, num_hiddens, num_outputs): super(LSTMNet, self).__init__() #nn.Conv1d(1,16,2), #nn.Sigmoid(), # nn.MaxPool1d(2), #nn.Conv1d(1,32,2), self.hidden_size = num_hiddens # RNN 层,这里的 batch_first 指定传入的是 (批大小,序列长度,序列每个位置的大小) # 如果不指定其为 True,传入顺序应当是 (序列长度,批大小,序列每个位置的大小) input_size= num_inputs.view(len(input_x), 1, -1)//24 self.rnn = torch.nn.LSTM(input_size, hidden_size=num_hiddens,batch_first=True) # 线性层 self.dense = torch.nn.Linear(self.hidden_size*24, 256) self.dense2 = torch.nn.Linear(256,num_outputs) # dropout 层,这里的参数指 dropout 的概率 self.dropout = torch.nn.Dropout(0.3) self.dropout2 = torch.nn.Dropout(0.5) # ReLU 层 self.relu = torch.nn.ReLU() # 前向传播函数,这是一个拼接的过程,使用大量变量是为了避免混淆,不做过多讲解 def forward(self, x): # x shape: (batch_size, 24, 307) # LSTM 层会传出其参数,这里用 _ 将其舍弃 h, _ = self.rnn(x) # LSTM 层会传出 (batch_size, 24, num_hiddens) 个参数,故需要 reshape 后丢入全连接层 h_r = h.reshape(-1,self.hidden_size*24) h_d = self.dropout(h_r) y = self.dense(h_d) drop_y = self.dropout2(y) a = self.relu(drop_y) y2 = self.dense2(a) return y2
这是一个使用LSTM网络进行序列预测的模型,输入是一个形状为(batch_size, 24, 307)的张量。在模型的初始化方法中,定义了LSTM层、线性层、dropout层和ReLU层。在前向传播方法中,首先将输入张量传入LSTM层得到输出h,然后将h重新reshape成形状为(batch_size, num_hiddens*24)的张量,再经过线性层、dropout层和ReLU层得到最终的预测结果y2。
阅读全文
相关推荐
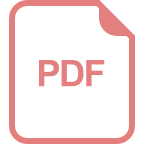
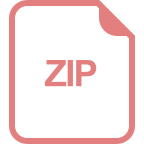











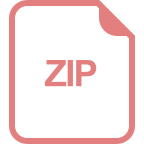


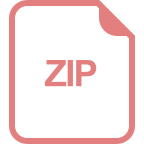
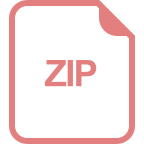