使用陀螺仪计算角度可移植的C语言代码
时间: 2023-06-02 15:02:18 浏览: 296
以下是使用陀螺仪计算角度的可移植的C语言代码示例:
```
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
// Gyroscope sensitivity in degrees per second
#define GYRO_SENSITIVITY 131.0
// Complementary filter constants
#define ALPHA 0.98
#define BETA 0.02
// Function to read raw gyro data
void read_gyro_raw(int16_t* gyro_x, int16_t* gyro_y, int16_t* gyro_z);
int main() {
// Initialize gyro data
int16_t gyro_x_raw, gyro_y_raw, gyro_z_raw;
float gyro_x, gyro_y, gyro_z;
float gyro_x_offset = 0.0, gyro_y_offset = 0.0, gyro_z_offset = 0.0;
// Initialize angle data
float angle_x = 0.0, angle_y = 0.0, angle_z = 0.0;
// Initialize time data
uint32_t prev_time = 0, curr_time = 0, delta_time = 0;
// Main loop
while (1) {
// Read gyro data
read_gyro_raw(&gyro_x_raw, &gyro_y_raw, &gyro_z_raw);
// Convert gyro data to degrees per second
gyro_x = gyro_x_raw / GYRO_SENSITIVITY;
gyro_y = gyro_y_raw / GYRO_SENSITIVITY;
gyro_z = gyro_z_raw / GYRO_SENSITIVITY;
// Apply gyro offsets
gyro_x -= gyro_x_offset;
gyro_y -= gyro_y_offset;
gyro_z -= gyro_z_offset;
// Calculate delta time
curr_time = millis(); // Replace with appropriate function
delta_time = curr_time - prev_time;
prev_time = curr_time;
// Calculate angle change from gyro data
angle_x += gyro_x * delta_time / 1000.0;
angle_y += gyro_y * delta_time / 1000.0;
angle_z += gyro_z * delta_time / 1000.0;
// Apply complementary filter to angle data
float accel_x, accel_y, accel_z;
// Read accelerometer data and convert to G's
// Replace with appropriate function
// ...
// Calculate angles from accelerometer data
accel_x = atan2(accel_y, accel_z) * 180.0 / PI;
accel_y = atan2(-accel_x, accel_z) * 180.0 / PI;
accel_z = 0.0;
// Apply complementary filter
angle_x = ALPHA * (angle_x + gyro_x * delta_time / 1000.0) + BETA * accel_x;
angle_y = ALPHA * (angle_y + gyro_y * delta_time / 1000.0) + BETA * accel_y;
angle_z = ALPHA * (angle_z + gyro_z * delta_time / 1000.0) + BETA * accel_z;
// Print angles
printf("Angle X: %.2f\n", angle_x);
printf("Angle Y: %.2f\n", angle_y);
printf("Angle Z: %.2f\n", angle_z);
}
return 0;
}
void read_gyro_raw(int16_t* gyro_x, int16_t* gyro_y, int16_t* gyro_z) {
// Replace with appropriate function to read raw gyro data
// ...
}
```
该代码使用了一个简单的组合滤波器将陀螺仪数据和加速度计数据相结合,以获得更准确的角度测量。代码中的一些常量需要根据实际硬件进行调整,例如陀螺仪灵敏度和滤波器常量。此外,代码中的一些函数需要根据实际硬件和平台进行替换。
相关推荐
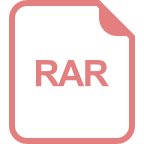
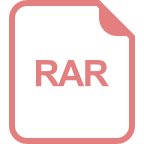














