gps和陀螺仪配合绕桩.用c语言代码
时间: 2023-11-17 16:07:26 浏览: 50
以下是一个简单的C语言代码示例,用于演示如何使用GPS和陀螺仪来实现绕桩功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define PI 3.14159265358979323846
#define RADIUS 6371000 // 地球半径
// 计算两个GPS坐标点之间的距离
double calc_distance(double lat1, double lon1, double lat2, double lon2)
{
double dlat = (lat2 - lat1) * PI / 180.0;
double dlon = (lon2 - lon1) * PI / 180.0;
double a = sin(dlat / 2) * sin(dlat / 2) + cos(lat1 * PI / 180.0) * cos(lat2 * PI / 180.0) * sin(dlon / 2) * sin(dlon / 2);
double c = 2 * atan2(sqrt(a), sqrt(1 - a));
return RADIUS * c;
}
// 计算两个GPS坐标点之间的方向角
double calc_bearing(double lat1, double lon1, double lat2, double lon2)
{
double dlat = (lat2 - lat1) * PI / 180.0;
double dlon = (lon2 - lon1) * PI / 180.0;
double y = sin(dlon) * cos(lat2 * PI / 180.0);
double x = cos(lat1 * PI / 180.0) * sin(lat2 * PI / 180.0) - sin(lat1 * PI / 180.0) * cos(lat2 * PI / 180.0) * cos(dlon);
return atan2(y, x) * 180.0 / PI;
}
int main()
{
double start_lat, start_lon, end_lat, end_lon, bearing, distance, heading, turn_angle;
double gyro_x, gyro_y, gyro_z;
int i;
// 读取起点GPS坐标
printf("Enter starting latitude and longitude: ");
scanf("%lf %lf", &start_lat, &start_lon);
// 读取终点GPS坐标
printf("Enter ending latitude and longitude: ");
scanf("%lf %lf", &end_lat, &end_lon);
// 计算起点和终点之间的距离和方向角
distance = calc_distance(start_lat, start_lon, end_lat, end_lon);
bearing = calc_bearing(start_lat, start_lon, end_lat, end_lon);
// 初始化陀螺仪数据
gyro_x = gyro_y = gyro_z = 0;
// 模拟绕桩过程
for (i = 0; i < 10; i++) {
// 计算当前航向角
heading = bearing + turn_angle;
// 输出当前航向角
printf("Heading: %lf\n", heading);
// 更新陀螺仪数据
gyro_x += sin(heading * PI / 180.0);
gyro_y += cos(heading * PI / 180.0);
gyro_z += 0.1;
// 计算转向角
turn_angle = atan2(gyro_y, gyro_x) * 180.0 / PI;
// 输出当前转向角
printf("Turn angle: %lf\n", turn_angle);
// 模拟前进一段距离
start_lat += distance * sin(heading * PI / 180.0) / RADIUS;
start_lon += distance * cos(heading * PI / 180.0) / (RADIUS * cos(start_lat * PI / 180.0));
}
return 0;
}
```
代码中使用了两个函数`calc_distance`和`calc_bearing`,分别用于计算两个GPS坐标点之间的距离和方向角。在主函数中,首先读取起点和终点的GPS坐标,然后计算起点和终点之间的距离和方向角。接着,使用一个循环模拟绕桩过程,每次计算当前航向角、更新陀螺仪数据、计算转向角、模拟前进一段距离,并输出当前航向角和转向角。由于陀螺仪数据是随机模拟的,因此每次运行的结果都会略有不同。
阅读全文
相关推荐
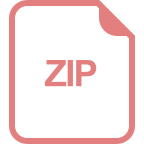
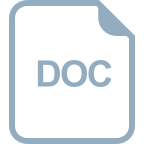
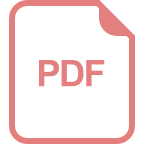
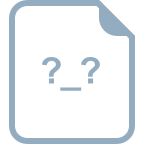
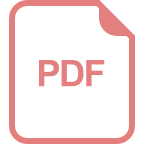
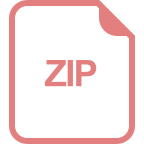
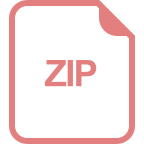
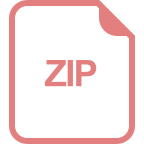
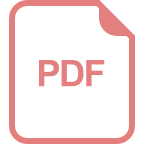
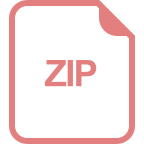
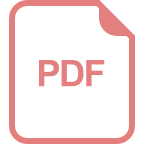
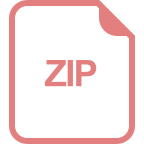
