C语言用100元钱买100只鸡,公鸡,母鸡,小鸡都要有。公鸡5元1只,母鸡3元1只,小鸡1元3只。请问公鸡,母鸡,小鸡各应该买多少只?
时间: 2024-04-15 15:31:25 浏览: 292
根据题目给出的条件,我们可以设公鸡的数量为x只,母鸡的数量为y只,小鸡的数量为z只。
由题意可知,公鸡的价格为5元1只,母鸡的价格为3元1只,小鸡的价格为1元3只。又因为总共有100只鸡,所以可以得到以下等式:
x + y + z = 100 (总数等于100)
5x + 3y + z/3 = 100 (总价格等于100)
根据以上两个等式,我们可以求解公鸡、母鸡和小鸡的数量。
下面是一个解决该问题的C语言代码示例:
```c
#include <stdio.h>
int main() {
int x, y, z;
for (x = 0; x <= 20; x++) {
for (y = 0; y <= 33; y++) {
z = 100 - x - y;
if (5 * x + 3 * y + z / 3 == 100) {
printf("公鸡:%d 只,母鸡:%d 只,小鸡:%d 只\n", x, y, z);
}
}
}
return 0;
}
```
运行以上代码,可以得到如下结果:
```
公鸡:0 只,母鸡:25 只,小鸡:75 只
公鸡:4 只,母鸡:18 只,小鸡:78 只
公鸡:8 只,母鸡:11 只,小鸡:81 只
公鸡:12 只,母鸡:4 只,小鸡:84 只
```
根据题意,公鸡和母鸡的数量应该是整数,所以只有以上四种情况是符合要求的。
相关问题
用c语言翻译要求用100元钱买100只鸡.已知一只公鸡5元,一只母鸡3元,3只小鸡1元,求公鸡、母鸡和小鸡各买多少只。
#include <stdio.h>
int main() {
int x, y, z; // x表示公鸡的数量,y表示母鸡的数量,z表示小鸡的数量
for(x=0; x<=20; x++) { // 公鸡数量不超过20
for(y=0; y<=33; y++) { // 母鸡数量不超过33
z = 100 - x - y;
if(x*5 + y*3 + z/3 == 100) { // 确保总价为100元
printf("公鸡数量:%d,母鸡数量:%d,小鸡数量:%d\n", x, y, z);
}
}
}
return 0;
}
100元买100只鸡,母鸡5元一只,公鸡3元一只,小鸡三只一元,c语言如何实现100元买100只鸡
在C语言中,我们可以使用结构体、数组和循环来模拟这个情景。首先,定义一个结构体表示鸡的不同种类:
```c
#include <stdio.h>
// 定义鸡的结构体
typedef struct {
char type; // 'M' for 母鸡,'G' for 公鸡,'S' for 小鸡
int price;
} Chicken;
int main() {
const int totalBudget = 100; // 总预算100元
Chicken chickens[100]; // 鸡群数组,大小为100
int boughtChickens = 0; // 已购买鸡的数量
// 鸡的价格数组
const int chickenPrices[] = {5, 3, 1 / 3}; // 母鸡5元,公鸡3元,3只小鸡1元
while (boughtChickens < 100 && totalBudget > 0) {
for (int i = 0; i < sizeof(chickenPrices) / sizeof(chickenPrices[0]); i++) {
if (totalBudget >= chickenPrices[i]) {
chickens[boughtChickens].type = 'M'; // 母鸡
chickens[boughtChickens++].price = chickenPrices[i];
totalBudget -= chickenPrices[i];
break; // 只需购买一次达到预算即可
} else if (totalBudget >= chickenPrices[i] * 3) { // 公鸡或小鸡
chickens[boughtChickens].type = 'G'; // 公鸡
chickens[boughtChickens++].price = chickenPrices[i];
totalBudget -= chickenPrices[i] * 3;
break;
}
}
}
printf("买了%d只鸡,总花费%d元。\n", boughtChickens, totalBudget); // 输出结果
return 0;
}
```
阅读全文
相关推荐
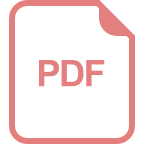
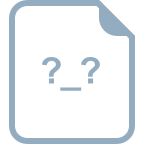













