数据结构Java版骑士游历问题
时间: 2023-07-06 08:41:59 浏览: 73
骑士游历问题是指给定一个起始点和目标点,求出骑士从起始点出发到达目标点的最短路线。这个问题可以使用深度优先搜索或广度优先搜索来解决。以下是使用广度优先搜索的Java代码实现。
```java
import java.util.LinkedList;
import java.util.Queue;
public class KnightTour {
private static final int BOARD_SIZE = 8;
private static final int[] ROW_MOVES = {-2, -1, 1, 2, 2, 1, -1, -2};
private static final int[] COL_MOVES = {1, 2, 2, 1, -1, -2, -2, -1};
public static void main(String[] args) {
int[][] board = new int[BOARD_SIZE][BOARD_SIZE];
int startX = 0;
int startY = 0;
int endX = 7;
int endY = 7;
int steps = findShortestPath(board, startX, startY, endX, endY);
System.out.println("Minimum number of steps required: " + steps);
}
private static int findShortestPath(int[][] board, int startX, int startY, int endX, int endY) {
Queue<Node> queue = new LinkedList<>();
queue.offer(new Node(startX, startY, 0));
board[startX][startY] = 1;
while (!queue.isEmpty()) {
Node node = queue.poll();
int x = node.x;
int y = node.y;
int steps = node.steps;
if (x == endX && y == endY) {
return steps;
}
for (int i = 0; i < ROW_MOVES.length; i++) {
int nextX = x + ROW_MOVES[i];
int nextY = y + COL_MOVES[i];
if (nextX >= 0 && nextX < BOARD_SIZE && nextY >= 0 && nextY < BOARD_SIZE && board[nextX][nextY] == 0) {
board[nextX][nextY] = 1;
queue.offer(new Node(nextX, nextY, steps + 1));
}
}
}
return -1;
}
private static class Node {
int x;
int y;
int steps;
public Node(int x, int y, int steps) {
this.x = x;
this.y = y;
this.steps = steps;
}
}
}
```
在这个实现中,我们使用一个二维数组 `board` 来标记每个位置是否被访问过。我们使用一个队列 `queue` 来存储待访问的节点。开始时,我们将起始节点加入队列中并标记为已访问。然后,我们从队列中取出一个节点,并尝试在周围的八个位置中找到未被访问过的位置。如果找到了目标位置,则返回当前步数。否则,将找到的位置加入队列中并标记为已访问,然后继续搜索。
注意,我们使用了一个 `Node` 类来表示每个节点的坐标和步数。这样可以更方便地进行节点的处理和存储。
相关推荐
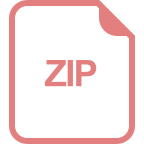
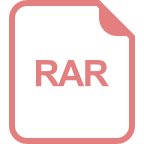
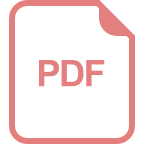














