Java语言用队列骑士游历问题
时间: 2023-09-16 13:10:53 浏览: 86
可以通过广度优先搜索算法来解决队列骑士游历问题。具体地,将每一个可能的下一步位置作为扩展节点加入到搜索队列中,直到找到目标位置为止。这个过程中需要考虑每个位置是否合法以及是否已经被访问过,以避免重复搜索。在Java语言中,可以使用Queue接口来实现队列,使用HashSet或者HashMap来存储已经访问过的节点,从而实现队列骑士游历问题的解决。
相关问题
java用队列实现骑士游历问题
骑士游历问题是一个经典的图论问题,可以使用广度优先搜索算法和队列来解决。
具体实现过程如下:
1. 定义一个二维数组来表示棋盘,棋盘的每个位置用一个整数表示,初始值为0,表示该位置还未被访问过。
2. 定义一个队列,用来存储待访问的节点。
3. 定义一个数组来表示骑士移动的方向,例如:int[][] directions = {{-2, -1}, {-1, -2}, {1, -2}, {2, -1}, {2, 1}, {1, 2}, {-1, 2}, {-2, 1}};。
4. 从棋盘的任意一个位置开始,将该位置入队,并将其值设为1,表示已经访问过。
5. 对队列进行循环操作,每次取出队首的节点,遍历其所有可能的移动方向,如果移动后的位置仍在棋盘内且未被访问过,则将该位置入队,并将其值设为当前节点的值加1。
6. 重复步骤5,直到队列为空或者找到目标位置。
7. 如果找到了目标位置,从目标位置开始倒推,直到回到起点,得到骑士游历的路径。
下面是Java代码示例:
```java
import java.util.*;
public class KnightTour {
private int[][] board;
private int size;
private int[][] directions = {{-2, -1}, {-1, -2}, {1, -2}, {2, -1}, {2, 1}, {1, 2}, {-1, 2}, {-2, 1}};
public KnightTour(int size) {
this.size = size;
board = new int[size][size];
}
public boolean solve(int startX, int startY, int endX, int endY) {
if (startX < 0 || startX >= size || startY < 0 || startY >= size
|| endX < 0 || endX >= size || endY < 0 || endY >= size) {
return false;
}
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startX, startY});
board[startX][startY] = 1;
while (!queue.isEmpty()) {
int[] curr = queue.poll();
if (curr[0] == endX && curr[1] == endY) {
return true;
}
for (int[] direction : directions) {
int nextX = curr[0] + direction[0];
int nextY = curr[1] + direction[1];
if (nextX >= 0 && nextX < size && nextY >= 0 && nextY < size && board[nextX][nextY] == 0) {
board[nextX][nextY] = board[curr[0]][curr[1]] + 1;
queue.offer(new int[]{nextX, nextY});
}
}
}
return false;
}
public List<int[]> getPath(int startX, int startY, int endX, int endY) {
if (!solve(startX, startY, endX, endY)) {
return null;
}
List<int[]> path = new ArrayList<>();
int[] curr = {endX, endY};
path.add(curr);
while (curr[0] != startX || curr[1] != startY) {
for (int[] direction : directions) {
int prevX = curr[0] - direction[0];
int prevY = curr[1] - direction[1];
if (prevX >= 0 && prevX < size && prevY >= 0 && prevY < size && board[prevX][prevY] == board[curr[0]][curr[1]] - 1) {
curr = new int[]{prevX, prevY};
path.add(curr);
break;
}
}
}
Collections.reverse(path);
return path;
}
public static void main(String[] args) {
KnightTour kt = new KnightTour(8);
List<int[]> path = kt.getPath(0, 0, 7, 7);
if (path == null) {
System.out.println("No solution");
} else {
for (int[] pos : path) {
System.out.println(pos[0] + " " + pos[1]);
}
}
}
}
```
使用java栈和队列解决骑士游历问题
骑士游历问题是指在一个给定的棋盘上,骑士从任意一个方格出发,只能按照“日”字型的步伐进行移动,直到覆盖棋盘上的每一个方格,求出骑士的移动路径。
这个问题可以使用深度优先搜索(DFS)和广度优先搜索(BFS)算法来解决。而栈和队列则可以用来实现DFS和BFS算法。
下面是使用java栈和队列解决骑士游历问题的代码示例:
```java
import java.util.*;
public class KnightTour {
private static int[][] board;
private static int[] dx = {-2, -1, 1, 2, 2, 1, -1, -2};
private static int[] dy = {1, 2, 2, 1, -1, -2, -2, -1};
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入棋盘大小:");
int n = scanner.nextInt();
board = new int[n][n];
System.out.print("请输入起始位置x坐标:");
int startX = scanner.nextInt();
System.out.print("请输入起始位置y坐标:");
int startY = scanner.nextInt();
System.out.println("BFS算法求解:");
bfs(startX, startY, n);
System.out.println();
System.out.println("DFS算法求解:");
dfs(startX, startY, n);
System.out.println();
}
// BFS算法
private static void bfs(int x, int y, int n) {
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{x, y});
board[x][y] = 1;
while (!queue.isEmpty()) {
int[] cur = queue.poll();
int cx = cur[0], cy = cur[1];
System.out.println("(" + cx + "," + cy + ")");
for (int i = 0; i < 8; i++) {
int nx = cx + dx[i], ny = cy + dy[i];
if (nx >= 0 && nx < n && ny >= 0 && ny < n && board[nx][ny] == 0) {
queue.offer(new int[]{nx, ny});
board[nx][ny] = board[cx][cy] + 1;
}
}
}
}
// DFS算法
private static void dfs(int x, int y, int n) {
Stack<int[]> stack = new Stack<>();
stack.push(new int[]{x, y});
board[x][y] = 1;
while (!stack.isEmpty()) {
int[] cur = stack.pop();
int cx = cur[0], cy = cur[1];
System.out.println("(" + cx + "," + cy + ")");
for (int i = 0; i < 8; i++) {
int nx = cx + dx[i], ny = cy + dy[i];
if (nx >= 0 && nx < n && ny >= 0 && ny < n && board[nx][ny] == 0) {
stack.push(new int[]{nx, ny});
board[nx][ny] = board[cx][cy] + 1;
}
}
}
}
}
```
在上面的代码中,我们定义了一个`board`二维数组来表示棋盘,其中0表示未走过的格子,1表示起始位置,2表示第二步,以此类推。`dx`和`dy`数组分别表示骑士的8种移动方式。`bfs`方法和`dfs`方法分别使用队列和栈来实现BFS和DFS算法。使用`System.out.println`打印出每一个移动的位置,最终可以得到骑士的移动路径。
阅读全文
相关推荐
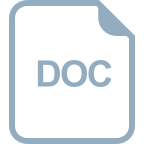
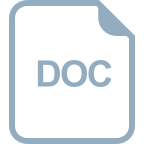
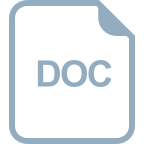


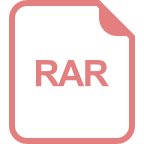



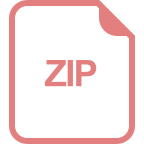
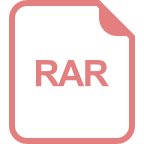



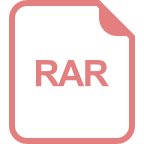
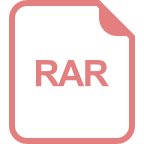