python 实现图像的无损压缩代码
时间: 2023-07-23 16:16:05 浏览: 113
图像的无损压缩可以使用PNG格式实现,PNG使用了无损压缩算法,能够保证图像质量不受影响,下面是一个Python实现的示例代码:
```python
from PIL import Image
def compress_image(infile, outfile):
try:
with Image.open(infile) as im:
# 使用PNG格式保存图片
im.save(outfile, "PNG")
print("图片压缩成功!")
except Exception as e:
print("图片压缩失败!", e)
if __name__ == '__main__':
infile = "test.jpg"
outfile = "test_compressed.png"
compress_image(infile, outfile)
```
这个例子使用了Pillow库,首先打开要压缩的图片,然后使用PNG格式保存图片,保存后的图片就是经过无损压缩的。你可以将`test.jpg`替换成你想要压缩的图片路径,将`test_compressed.png`替换成你想要保存的压缩后的图片路径。
相关问题
python 实现批量图像的无损压缩
以下是Python实现的批量图像的无损压缩示例代码:
```python
from PIL import Image
import os
def compress_images(input_dir, output_dir):
for root, dirs, files in os.walk(input_dir):
for file in files:
infile = os.path.join(root, file)
outfile = os.path.join(output_dir, file.split(".")[0] + ".png")
try:
with Image.open(infile) as im:
# 使用PNG格式保存图片
im.save(outfile, "PNG")
print("图片压缩成功!", file)
except Exception as e:
print("图片压缩失败!", file, e)
if __name__ == '__main__':
input_dir = "input"
output_dir = "output"
compress_images(input_dir, output_dir)
```
这个例子使用了Pillow库和os库,遍历指定的输入目录下的所有图片,将图片保存为PNG格式,保存到指定的输出目录下。你可以将`input`替换成你想要压缩的图片所在的目录,将`output`替换成你想要保存压缩后的图片的目录。
Python实现对原始图像的无损压缩
Python有很多库可以实现对原始图像的无损压缩,其中比较常用的是Python Imaging Library (PIL)和OpenCV。
下面以PIL库为例,介绍如何实现对原始图像的无损压缩:
```python
from PIL import Image
# 打开原始图像
image = Image.open("original_image.png")
# 保存为PNG格式,压缩级别为0(无损压缩)
image.save("compressed_image.png", format="PNG", compress_level=0)
```
在上述代码中,`Image.open()`方法用于打开原始图像,`Image.save()`方法用于保存压缩后的图像。在这里,我们将压缩级别设置为0,表示不进行压缩,即使用无损压缩方式。
需要注意的是,无损压缩并不一定能够减小图像文件的大小,因为它只是尽可能地减小图像文件的信息冗余,保证图像质量不受影响。如果需要进一步减小图像文件的大小,可以考虑使用有损压缩方式,但这样会降低图像质量。
除了PIL库,还有其他库如OpenCV也可以实现对原始图像的无损压缩,使用方法类似,只是具体的函数名称和参数可能会有所不同。
阅读全文
相关推荐
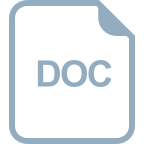
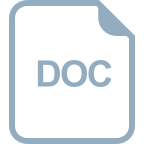
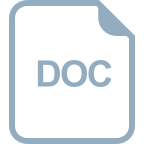
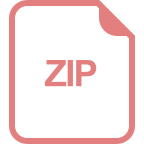
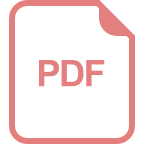
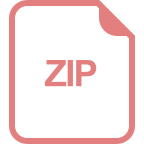
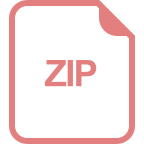
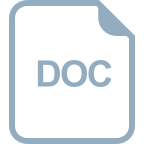
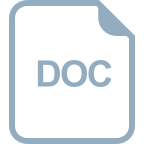
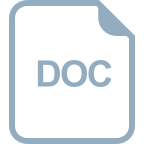





