用java编写一个类,类中包含下列类方法:1)判断一个整数是否是素数的方法;2)判断一个整数是否是回文的方法;3)求一个正整数的阶乘的方法(此方法使用递归求阶乘);4)求一个正整数的逆序数的方法。然后再编写一个测试类,调用这些方法进行验证。
时间: 2024-03-03 18:47:58 浏览: 96
好的,以下是Java代码实现:
```java
public class MathUtils {
// 判断一个整数是否是素数的方法
public static boolean isPrime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i <= Math.sqrt(n); i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
// 判断一个整数是否是回文的方法
public static boolean isPalindrome(int n) {
int reversed = 0;
int num = n;
while (num > 0) {
reversed = reversed * 10 + num % 10;
num /= 10;
}
return n == reversed;
}
// 求一个正整数的阶乘的方法(此方法使用递归求阶乘)
public static int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
// 求一个正整数的逆序数的方法
public static int reverse(int n) {
int reversed = 0;
while (n > 0) {
reversed = reversed * 10 + n % 10;
n /= 10;
}
return reversed;
}
}
```
测试类:
```java
public class MathUtilsTest {
public static void main(String[] args) {
// 测试isPrime方法
System.out.println(MathUtils.isPrime(7)); // true
System.out.println(MathUtils.isPrime(8)); // false
// 测试isPalindrome方法
System.out.println(MathUtils.isPalindrome(121)); // true
System.out.println(MathUtils.isPalindrome(123)); // false
// 测试factorial方法
System.out.println(MathUtils.factorial(5)); // 120
// 测试reverse方法
System.out.println(MathUtils.reverse(12345)); // 54321
}
}
```
输出结果:
```
true
false
true
false
120
54321
```
阅读全文
相关推荐







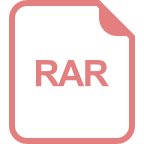

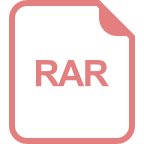


