stream ends prematurely at 22, should be 18446744073709551615
时间: 2024-04-21 22:30:28 浏览: 25
这个问题看起来像是出现在数据传输或处理过程中的错误信息。"stream ends prematurely" 意味着数据流在预期之前结束了,而 "should be 18446744073709551615" 则表明它预计的长度应该是一个非常大的数字。
这种情况可能是由于数据传输中断、数据格式错误、数据丢失或其他问题导致的。如果您能提供更多上下文信息,例如出现错误的环境、数据来源、数据格式等,我就可以更好地帮助您解决这个问题。
相关问题
● A menu including Open and Exit where Open starts a JFileChooser to select the file with the questions inside and Exit ends the programme. ● Once a file is loaded, the GUI should display one question and its answers at a time. ● The user should be able to select an answer and they should be informed if they were correct or not. ● The user should be made aware of the number of correctly answered and the total number of questions answered. ● The user should only be able to proceed to the next question once they answered the current one. ● Once all questions have been answered, the user should be informed of their overall score and that the game has finished. The Open menu item should now be enabled to start a new quiz. Optionally, you can add a restart menu item to redo the current quiz.
下面是一个实现上述功能的Java Swing程序:
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.SwingConstants;
public class QuizGame implements ActionListener {
private JFrame frame;
private JLabel questionLabel;
private List<Question> questions;
private int currentQuestionIndex;
private int numCorrect;
private int numAnswered;
private JRadioButton[] answerButtons;
private JButton nextButton;
private JButton restartButton;
private JFileChooser fileChooser;
public QuizGame() {
frame = new JFrame("Quiz Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
JPanel questionPanel = new JPanel();
questionPanel.setLayout(new BorderLayout());
questionLabel = new JLabel();
questionLabel.setHorizontalAlignment(SwingConstants.CENTER);
questionPanel.add(questionLabel, BorderLayout.CENTER);
JPanel answerPanel = new JPanel();
answerPanel.setLayout(new BorderLayout());
JPanel buttonPanel = new JPanel();
nextButton = new JButton("Next");
nextButton.addActionListener(this);
buttonPanel.add(nextButton);
restartButton = new JButton("Restart");
restartButton.addActionListener(this);
buttonPanel.add(restartButton);
answerPanel.add(buttonPanel, BorderLayout.SOUTH);
answerButtons = new JRadioButton[4];
for (int i = 0; i < answerButtons.length; i++) {
answerButtons[i] = new JRadioButton();
answerPanel.add(answerButtons[i], BorderLayout.NORTH);
}
frame.add(questionPanel, BorderLayout.CENTER);
frame.add(answerPanel, BorderLayout.SOUTH);
fileChooser = new JFileChooser();
fileChooser.setFileSelectionMode(JFileChooser.FILES_ONLY);
questions = new ArrayList<Question>();
currentQuestionIndex = -1;
numCorrect = 0;
numAnswered = 0;
setMenu();
setNextEnabled(false);
setFrameVisible(true);
}
private void setMenu() {
MenuBar menuBar = new MenuBar();
Menu fileMenu = new Menu("File");
MenuItem openItem = new MenuItem("Open");
openItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int result = fileChooser.showOpenDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(";");
String question = parts[0];
String[] answers = parts[1].split(",");
String correctAnswer = answers[0];
Question q = new Question(question, answers, correctAnswer);
questions.add(q);
}
reader.close();
Collections.shuffle(questions);
currentQuestionIndex = -1;
numCorrect = 0;
numAnswered = 0;
setNextEnabled(true);
nextQuestion();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
});
MenuItem exitItem = new MenuItem("Exit");
exitItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
fileMenu.add(openItem);
fileMenu.add(exitItem);
menuBar.add(fileMenu);
frame.setMenuBar(menuBar);
}
private void setFrameVisible(boolean visible) {
frame.setVisible(visible);
}
private void setNextEnabled(boolean enabled) {
nextButton.setEnabled(enabled);
}
private void setRestartEnabled(boolean enabled) {
restartButton.setEnabled(enabled);
}
private void nextQuestion() {
currentQuestionIndex++;
if (currentQuestionIndex < questions.size()) {
Question q = questions.get(currentQuestionIndex);
questionLabel.setText(q.getQuestion());
String[] answers = q.getAnswers();
Collections.shuffle(Arrays.asList(answers));
for (int i = 0; i < answerButtons.length; i++) {
answerButtons[i].setText(answers[i]);
answerButtons[i].setSelected(false);
}
} else {
double score = (double) numCorrect / numAnswered;
String message = "You answered " + numCorrect + " out of " + numAnswered + " questions correctly.\n";
if (score >= 0.9) {
message += "Excellent!";
} else if (score >= 0.7) {
message += "Good job!";
} else if (score >= 0.5) {
message += "Not bad.";
} else {
message += "You need more practice.";
}
questionLabel.setText(message);
setNextEnabled(false);
setRestartEnabled(true);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == nextButton) {
if (currentQuestionIndex >= 0) {
Question q = questions.get(currentQuestionIndex);
numAnswered++;
boolean correct = false;
for (int i = 0; i < answerButtons.length; i++) {
JRadioButton button = answerButtons[i];
if (button.isSelected()) {
String answer = button.getText();
if (answer.equals(q.getCorrectAnswer())) {
correct = true;
numCorrect++;
}
break;
}
}
String message = correct ? "Correct!" : "Incorrect!";
questionLabel.setText(message);
}
setNextEnabled(false);
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
nextQuestion();
}
});
} else if (e.getSource() == restartButton) {
currentQuestionIndex = -1;
numCorrect = 0;
numAnswered = 0;
setRestartEnabled(false);
setNextEnabled(true);
nextQuestion();
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
QuizGame game = new QuizGame();
}
});
}
private class Question {
private String question;
private String[] answers;
private String correctAnswer;
public Question(String question, String[] answers, String correctAnswer) {
this.question = question;
this.answers = answers;
this.correctAnswer = correctAnswer;
}
public String getQuestion() {
return question;
}
public String[] getAnswers() {
return answers;
}
public String getCorrectAnswer() {
return correctAnswer;
}
}
}
```
这个程序使用了Java Swing的JFrame、JLabel、JRadioButton、JButton和JFileChooser来实现一个简单的问答游戏。在菜单中,使用JFileChooser选择包含问题和答案的文件,并将它们加载到List<Question>中。在游戏过程中,使用currentQuestionIndex跟踪当前问题的索引,并使用numCorrect和numAnswered跟踪正确回答的问题的数量和总问题数量。使用JRadioButton显示答案选项,并在用户选择一个选项后检查它是否正确,并显示相应的消息。使用nextButton和restartButton在用户回答问题后控制游戏的流程。最后,使用JOptionPane显示用户的总体分数和游戏结束的消息。
data segment table dw 1234h data ends
data segment table dw 1234h data ends 是一段汇编语言的指令。该指令使用了数据段定义符“dw”,并在数据段表中定义了一个变量。变量名为"data",初始值为1234h。
数据段表是一种记录数据段信息的数据结构。它通常由编译器或连接器生成,并用于管理程序中的不同数据段。数据段表中的每个条目都描述了一个数据段的起始地址、长度等信息。在该指令中,数据段表的条目为data segment table dw 1234h。
指令末尾的"data ends"表示数据段的结束。这个指令告诉编译器或连接器数据段定义的结束位置。这样,编译器或连接器能够正确地将数据段与程序的其他部分链接起来。
总结起来,该指令定义了一个数据段表条目,其中包含一个名为"data"的变量,初始值为1234h。同时,指令使用"data ends"表示数据段定义的结束位置。这样,在编译或链接过程中,编译器或连接器能够正确处理数据段。
相关推荐
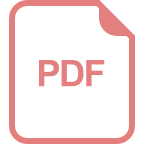
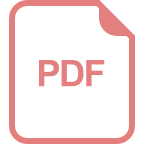












