Given an array of ints, return a new array length 2 containing the first and last elements from the original array. The original array will be length 1 or more. make_ends([1, 2, 3]) → [1, 3] make_ends([1, 2, 3, 4]) → [1, 4] make_ends([7, 4, 6, 2]) → [7, 2]
时间: 2023-07-02 09:13:47 浏览: 121
以下是一个 Python 函数,它可以接受一个整数列表作为参数,并返回一个包含原始列表的第一个和最后一个元素的新列表:
```python
def make_ends(nums):
return [nums[0], nums[-1]]
```
该函数首先使用 `nums[0]` 来获取列表的第一个元素,接着使用 `nums[-1]` 来获取列表的最后一个元素。最后,将这两个元素放入一个新列表中并返回该列表。
这个函数假设原始列表至少有一个元素。如果原始列表是空的,或只有一个元素,那么该函数将引发一个 `IndexError` 异常。如果需要,可以在函数中添加一些额外的代码来处理这些情况。
相关问题
Given an array of ints, return a new array length 2 containing the first and last elements from the original array. The original array will be length 1 or more.
Sure, here's the solution in Python:
```python
def make_ends(nums):
return [nums[0], nums[-1]]
```
This function takes an input array `nums` and returns a new array containing the first and last elements of the input array. We use the `[0]` index to access the first element of the array, and the `[-1]` index to access the last element of the array.
Given 2 int arrays, a and b, each length 3, return a new array length 2 containing their middle elements.
以下是 Python 代码实现:
```python
def middle_way(a, b):
return [a[1], b[1]]
```
这个函数接受两个长度为 3 的整数数组 `a` 和 `b` 作为输入,返回一个新的长度为 2 的数组,其中包含两个输入数组的中间元素。
例如,`middle_way([1, 2, 3], [4, 5, 6])` 的返回值为 `[2, 5]`。
阅读全文
相关推荐
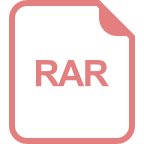
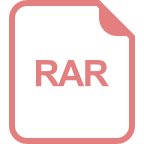
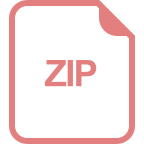











