""" Data class containing information about the detections in a video frame. Attributes: xyxy (np.ndarray): An array of shape `(n, 4)` containing the bounding boxes coordinates in format `[x1, y1, x2, y2]` mask: (Optional[np.ndarray]): An array of shape `(n, W, H)` containing the segmentation masks. confidence (Optional[np.ndarray]): An array of shape `(n,)` containing the confidence scores of the detections. class_id (Optional[np.ndarray]): An array of shape `(n,)` containing the class ids of the detections. tracker_id (Optional[np.ndarray]): An array of shape `(n,)` containing the tracker ids of the detections. """
时间: 2024-04-17 19:27:36 浏览: 22
这段代码描述了一个名为 `VideoFrameDetections` 的数据类,它包含有关视频帧中检测结果的信息。
该类具有以下属性:
- `xyxy`:一个形状为 `(n, 4)` 的数组,其中 `n` 是检测框的数量,包含了检测框的坐标信息,格式为 `[x1, y1, x2, y2]`。
- `mask`:可选属性,一个形状为 `(n, W, H)` 的数组,其中 `n` 是检测框的数量,`W` 和 `H` 是分割掩码的宽度和高度。它包含了分割掩码的信息。
- `confidence`:可选属性,一个形状为 `(n,)` 的数组,其中 `n` 是检测框的数量,包含了检测结果的置信度得分。
- `class_id`:可选属性,一个形状为 `(n,)` 的数组,其中 `n` 是检测框的数量,包含了检测结果的类别标签。
- `tracker_id`:可选属性,一个形状为 `(n,)` 的数组,其中 `n` 是检测框的数量,包含了检测结果的追踪器ID。
这个类提供了一种方便地组织和存储视频帧中的检测结果的方式。你可以使用这些属性来访问和操作检测结果的各个部分。如果还有其他问题,请随时提问。
相关问题
class PolygonZone: """ A class for defining a polygon-shaped zone within a frame for detecting objects. Attributes: polygon (np.ndarray): A polygon represented by a numpy array of shape `(N, 2)`, containing the `x`, `y` coordinates of the points. frame_resolution_wh (Tuple[int, int]): The frame resolution (width, height) triggering_position (Position): The position within the bounding box that triggers the zone (default: Position.BOTTOM_CENTER) current_count (int): The current count of detected objects within the zone mask (np.ndarray): The 2D bool mask for the polygon zone """ def __init__( self, polygon: np.ndarray, frame_resolution_wh: Tuple[int, int], triggering_position: Position = Position.BOTTOM_CENTER, ): self.polygon = polygon.astype(int) self.frame_resolution_wh = frame_resolution_wh self.triggering_position = triggering_position self.current_count = 0 width, height = frame_resolution_wh self.mask = polygon_to_mask( polygon=polygon, resolution_wh=(width + 1, height + 1) )
这是一个名为 `PolygonZone` 的类,用于定义帧中的多边形区域,用于检测对象。
该类具有以下属性:
- `polygon`:一个形状为 `(N, 2)` 的numpy数组,表示由 `x` 和 `y` 坐标组成的多边形的点。
- `frame_resolution_wh`:帧的分辨率(宽度,高度)的元组。
- `triggering_position`:触发区域内的位置(默认为 Position.BOTTOM_CENTER)。
- `current_count`:区域内检测到的对象的当前计数。
- `mask`:多边形区域的二维布尔掩码。
`PolygonZone` 类有一个初始化方法 `__init__`,它接受多边形、帧分辨率和触发位置作为参数。在初始化过程中,它将多边形转换为整数类型,并根据分辨率生成一个二维掩码。
以下是代码示例:
```python
import numpy as np
from typing import Tuple
class PolygonZone:
def __init__(
self,
polygon: np.ndarray,
frame_resolution_wh: Tuple[int, int],
triggering_position: Position = Position.BOTTOM_CENTER,
):
self.polygon = polygon.astype(int)
self.frame_resolution_wh = frame_resolution_wh
self.triggering_position = triggering_position
self.current_count = 0
width, height = frame_resolution_wh
self.mask = polygon_to_mask(
polygon=polygon, resolution_wh=(width + 1, height + 1)
)
```
请注意,上述代码中的 `Position` 类没有给出,您可能需要根据您的需要定义和实现它。此外,还缺少 `polygon_to_mask` 函数的实现。您需要根据您的需求自行实现该函数,以将多边形转换为掩码。
java.lang.AssertionError: Expected test to throw (an instance of android.os.RemoteException and exception with message a string containing "RemoteException")
这个错误通常出现在JUnit测试中,表示测试没有抛出预期的异常。在本例中,测试期望抛出一个android.os.RemoteException,并且异常消息中包含"RemoteException"字符串。
如果您遇到这个错误,可以尝试以下方法来解决问题:
1. 确保您的测试代码中包含了期望抛出异常的代码,并且异常确实被抛出了。如果您的代码没有抛出期望的异常,那么测试就会失败。
2. 确保您的测试代码中使用了正确的异常类型和异常消息。如果您期望抛出android.os.RemoteException,并且异常消息中包含"RemoteException"字符串,那么您的测试代码应该类似于下面的代码:
```java
@Test(expected = RemoteException.class)
public void testSomeMethod() throws RemoteException {
// Some code that throws a RemoteException...
throw new RemoteException("RemoteException occurred.");
}
```
在这个示例代码中,我们使用`@Test(expected = RemoteException.class)`注解来告诉JUnit测试我们期望抛出一个RemoteException异常。然后,我们在测试方法中抛出了一个带有异常消息的RemoteException异常。
3. 检查您的代码是否正确处理了异常。如果您的代码在抛出异常后没有正确处理它,那么测试就会失败。在这种情况下,您需要检查并修复代码中的错误。
总之,要解决这个错误,您需要仔细检查您的测试代码和被测试代码,确保它们正确处理异常,并使用正确的异常类型和异常消息。
相关推荐
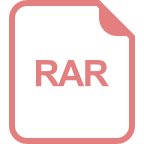
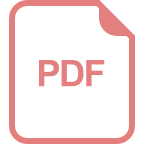
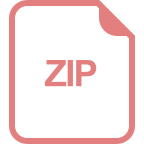











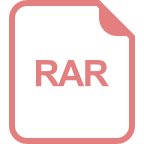
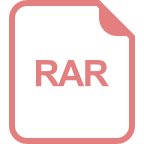