For this task you are required to write a class to represent a Dice. Your class should contain all features and functionality of a Die. Once you have your Dice class written you are going to write a very simple game. The game is one where each player has their own n sided Dice, and they win the game by being the first to reach a cumulative score after rolling their Dice. Your game should allow Dice the same n-sided dice for each player. The game should allow the number of players and the winning score to be decided by the user. It should print out the winning player the score they achieved and how many rolls it took them to achieve their score
时间: 2024-02-13 21:06:09 浏览: 82
Sure, I can help you with that. Here's an implementation of the Dice class:
```python
import random
class Dice:
def __init__(self, num_sides):
self.num_sides = num_sides
def roll(self):
return random.randint(1, self.num_sides)
```
This class has a constructor that takes in the number of sides of the dice and a roll method that returns a random number between 1 and the number of sides.
To create a simple game using this class, we can do the following:
```python
num_players = int(input("Enter the number of players: "))
num_sides = int(input("Enter the number of sides on the dice: "))
winning_score = int(input("Enter the winning score: "))
players = []
for i in range(num_players):
players.append({'id': i+1, 'score': 0, 'rolls': 0, 'dice': Dice(num_sides)})
winner = None
while not winner:
for player in players:
roll = player['dice'].roll()
player['score'] += roll
player['rolls'] += 1
print(f"Player {player['id']} rolled a {roll}. Current score: {player['score']}")
if player['score'] >= winning_score:
winner = player
break
print(f"\nPlayer {winner['id']} won with a score of {winner['score']} after {winner['rolls']} rolls.")
```
This code prompts the user for the number of players, the number of sides on the dice, and the winning score. It then creates a list of player dictionaries, each containing an ID, score, number of rolls, and a Dice object. The game loop iterates through each player, rolls their dice, updates their score and number of rolls, and checks if they have reached the winning score. If a player has reached the winning score, the game loop ends and the winner is announced.
阅读全文
相关推荐
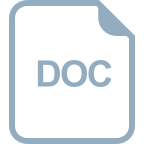
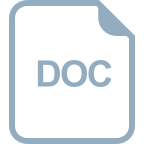
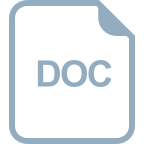
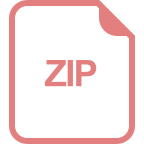












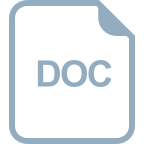
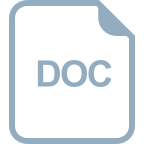
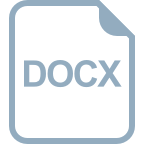