Implement the hash table (with chaining) data structure. Your table should be able to grow and shrink based on how many items are there. contain at least the following methods: * __init__: initialize the data structure * hash: implement a hash function that is chosen from a universal hash family * search: search for a key in the table, return True if found, False otherwise * insert: insert a key into the table if it is not already in there * delete: delete a key from the table * size: return the number of buckets in your hash table
时间: 2024-02-14 21:02:49 浏览: 79
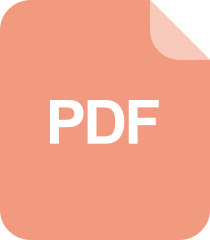
Swift.Data.Structure.and.Algorithms.2016.11.pdf
Sure, here's an implementation of a hash table with chaining in Python:
```python
import random
class HashTable:
def __init__(self, capacity=10):
self.capacity = capacity
self.size = 0
self.table = [[] for _ in range(self.capacity)]
def hash(self, key):
hash_value = 0
for i in range(len(key)):
hash_value = (hash_value * 31 + ord(key[i])) % self.capacity
return hash_value
def search(self, key):
hash_value = self.hash(key)
bucket = self.table[hash_value]
for i in range(len(bucket)):
if bucket[i][0] == key:
return True
return False
def insert(self, key):
if self.search(key):
return
hash_value = self.hash(key)
bucket = self.table[hash_value]
bucket.append((key, None))
self.size += 1
if self.size >= self.capacity * 0.7:
self._resize()
def delete(self, key):
hash_value = self.hash(key)
bucket = self.table[hash_value]
for i in range(len(bucket)):
if bucket[i][0] == key:
bucket.pop(i)
self.size -= 1
if self.size <= self.capacity * 0.3:
self._resize()
return
def size(self):
return self.capacity
def _resize(self):
self.capacity *= 2
new_table = [[] for _ in range(self.capacity)]
for bucket in self.table:
for key, value in bucket:
hash_value = self.hash(key)
new_table[hash_value].append((key, value))
self.table = new_table
```
The `__init__` method initializes the hash table with a specified capacity (default is 10), and creates a list of empty lists to represent the buckets.
The `hash` method takes in a key and maps it to an integer value between 0 and the capacity of the table using a hash function. In this case, we're using a simple polynomial rolling hash function.
The `search` method searches for a key in the table by computing its hash value and checking if it exists in the corresponding bucket.
The `insert` method inserts a key into the table by computing its hash value and appending it to the corresponding bucket. If the load factor of the table exceeds 0.7, the table is resized to double its capacity.
The `delete` method deletes a key from the table by computing its hash value and removing it from the corresponding bucket. If the load factor of the table falls below 0.3, the table is resized to half its capacity.
The `size` method simply returns the current capacity of the table.
The `_resize` method is a private helper method that is called by the `insert` and `delete` methods when the load factor threshold is exceeded. It creates a new table with double or half the capacity of the current table, and rehashes all the keys in the old table into the new table.
阅读全文
相关推荐
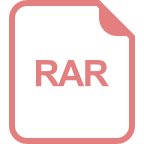
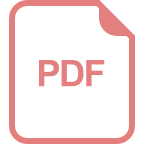















