单链表的有序排列C语言代码
时间: 2024-11-14 16:14:20 浏览: 3
在C语言中,单链表是一种线性的数据结构,通常用于存储一组按顺序排列的数据。这里有一个简单的示例,展示了如何通过比较节点值对单链表进行升序排序:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入节点到已排序链表
void insertSorted(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (*head == NULL || (*head)->data > value) {
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* head = NULL; // 初始链表为空
// 假设我们要插入的值
int values[] = {5, 2, 8, 1, 9};
for (int i = 0; i < sizeof(values) / sizeof(values[0]); i++) {
insertSorted(&head, values[i]);
}
printf("Sorted linked list: ");
printList(head);
return 0;
}
```
在这个例子中,`insertSorted`函数会将新元素插入到已排序链表的适当位置,而`printList`则用于展示排序后的链表。
阅读全文
相关推荐
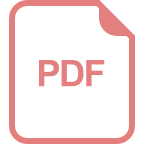
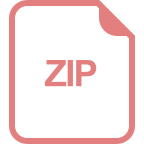















