unity quad shader 后 Shader "EH_SampleShader/ToonShader" { 保存图片到 temp.png 目录
时间: 2024-04-15 10:26:19 浏览: 103
要将Unity Quad使用的Shader渲染结果保存为图片,你可以使用RenderTexture和Texture2D来实现。以下是一个保存Quad渲染结果的示例代码:
```csharp
using UnityEngine;
public class SaveQuadRender : MonoBehaviour
{
public RenderTexture renderTexture;
public string savePath = "temp.png";
private void Start()
{
// 创建RenderTexture,并设置宽高与Quad相同
renderTexture = new RenderTexture(Screen.width, Screen.height, 24);
// 设置摄像机的渲染目标为renderTexture
Camera.main.targetTexture = renderTexture;
// 创建一张与renderTexture尺寸相同的Texture2D
Texture2D texture = new Texture2D(renderTexture.width, renderTexture.height, TextureFormat.RGBA32, false);
// 渲染摄像机
Camera.main.Render();
// 激活renderTexture
RenderTexture.active = renderTexture;
// 读取像素内容到texture
texture.ReadPixels(new Rect(0, 0, renderTexture.width, renderTexture.height), 0, 0);
texture.Apply();
// 将texture保存为图片文件
byte[] bytes = texture.EncodeToPNG();
System.IO.File.WriteAllBytes(savePath, bytes);
// 释放资源
RenderTexture.active = null;
Camera.main.targetTexture = null;
Destroy(renderTexture);
Destroy(texture);
}
}
```
在这个示例中,我们首先创建了一个与屏幕大小相同的RenderTexture,并将摄像机的渲染目标设置为这个RenderTexture。这样,Quad渲染的结果就会被渲染到这个RenderTexture上。
然后,我们创建了一个与RenderTexture尺寸相同的Texture2D,并使用ReadPixels函数从RenderTexture中读取像素内容。接着,我们调用Apply函数来应用像素更改。
最后,我们使用EncodeToPNG函数将Texture2D保存为PNG格式的字节数组,并使用WriteAllBytes函数将字节数组写入文件中,保存为temp.png。
请确保将这段代码添加到一个游戏对象上,并设置好保存路径savePath。当游戏运行时,Quad的渲染结果将保存为temp.png文件。
阅读全文
相关推荐
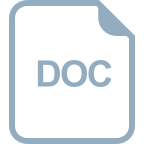
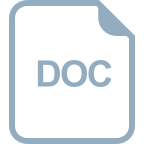
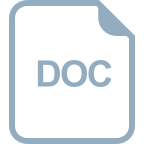


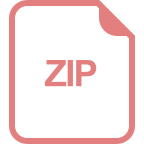
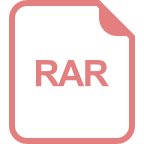
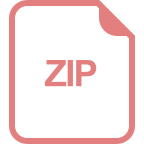
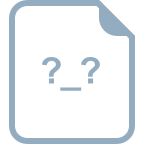
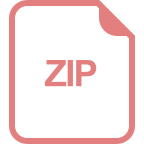
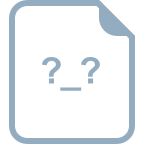
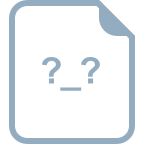
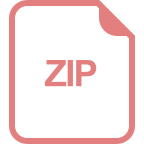
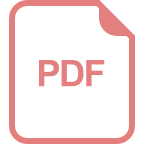
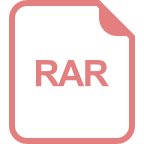
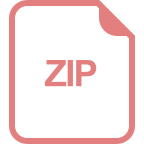
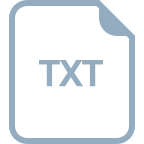
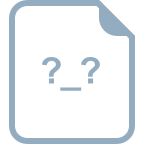