modes = stat.S_IWUSR | stat.S_IRUSR 需要导入什么包
时间: 2024-03-30 17:05:46 浏览: 20
对不起,我之前的回答有误。`stat.S_IWUSR` 和 `stat.S_IRUSR` 是 Python 中的常量,用于设置文件权限。这些常量属于 `stat` 模块,需要导入 `stat` 包才能使用。
正确的导入语句如下所示:
```python
import stat
```
这样,您就可以使用 `stat.S_IWUSR` 和 `stat.S_IRUSR` 这些常量来设置文件权限了。非常抱歉给您带来的困惑。
相关问题
modes = stat.S_IWUSR | stat.S_IRUSR # 注意根据具体业务的需要设置文件权限
您提到了 `modes = stat.S_IWUSR | stat.S_IRUSR`,这是一个设置文件权限的示例代码。`stat.S_IWUSR` 表示设置文件所有者的写权限,`stat.S_IRUSR` 表示设置文件所有者的读权限。通过使用位运算符 `|`,可以将这两个权限合并到 `modes` 变量中。
根据您的具体业务需求,您可以根据需要进行设置文件的权限。除了文件所有者的权限外,还可以使用 `stat.S_IWGRP`(文件所属组的写权限)、`stat.S_IRGRP`(文件所属组的读权限)、`stat.S_IWOTH`(其他用户的写权限)和 `stat.S_IROTH`(其他用户的读权限)等来设置不同类型用户的权限。根据实际情况,您可以按照需要组合这些权限,并将结果赋值给 `modes` 变量。
优化代码 def fault_classification_wrapper(vin, main_path, data_path, log_path, done_path): start_time = time.time() isc_path = os.path.join(done_path, vin, 'isc_cal_result', f'{vin}_report.xlsx') if not os.path.exists(isc_path): print('No isc detection input!') else: isc_input = isc_produce_alarm(isc_path, vin) ica_path = os.path.join(done_path, vin, 'ica_cal_result', f'ica_detection_alarm_{vin}.csv') if not os.path.exists(ica_path): print('No ica detection input!') else: ica_input = ica_produce_alarm(ica_path) soh_path = os.path.join(done_path, vin, 'SOH_cal_result', f'{vin}_sohAno.csv') if not os.path.exists(soh_path): print('No soh detection input!') else: soh_input = soh_produce_alarm(soh_path, vin) alarm_df = pd.concat([isc_input, ica_input, soh_input]) alarm_df.reset_index(drop=True, inplace=True) alarm_df['alarm_cell'] = alarm_df['alarm_cell'].apply(lambda _: str(_)) print(vin) module = AutoAnalysisMain(alarm_df, main_path, data_path, done_path) module.analysis_process() flags = os.O_WRONLY | os.O_CREAT modes = stat.S_IWUSR | stat.S_IRUSR with os.fdopen(os.open(os.path.join(log_path, 'log.txt'), flags, modes), 'w') as txt_file: for k, v in module.output.items(): txt_file.write(k + ':' + str(v)) txt_file.write('\n') for x, y in module.output_sub.items(): txt_file.write(x + ':' + str(y)) txt_file.write('\n\n') fc_result_path = os.path.join(done_path, vin, 'fc_result') if not os.path.exists(fc_result_path): os.makedirs(fc_result_path) pd.DataFrame(module.output).to_csv( os.path.join(fc_result_path, 'main_structure.csv')) df2 = pd.DataFrame() for subs in module.output_sub.keys(): sub_s = pd.Series(module.output_sub[subs]) df2 = df2.append(sub_s, ignore_index=True) df2.to_csv(os.path.join(fc_result_path, 'sub_structure.csv')) end_time = time.time() print("time cost of fault classification:", float(end_time - start_time) * 1000.0, "ms") return
Here are some suggestions to optimize the code:
1. Use list comprehension to simplify the code:
```
alarm_df = pd.concat([isc_input, ica_input, soh_input]).reset_index(drop=True)
alarm_df['alarm_cell'] = alarm_df['alarm_cell'].apply(str)
```
2. Use context manager to simplify file operation:
```
with open(os.path.join(log_path, 'log.txt'), 'w') as txt_file:
for k, v in module.output.items():
txt_file.write(f"{k}:{v}\n")
for x, y in module.output_sub.items():
txt_file.write(f"{x}:{y}\n\n")
```
3. Use `Pathlib` to simplify path operation:
```
fc_result_path = Path(done_path) / vin / 'fc_result'
fc_result_path.mkdir(parents=True, exist_ok=True)
pd.DataFrame(module.output).to_csv(fc_result_path / 'main_structure.csv')
pd.DataFrame(module.output_sub).to_csv(fc_result_path / 'sub_structure.csv')
```
4. Use f-string to simplify string formatting:
```
print(f"time cost of fault classification: {(end_time - start_time) * 1000.0} ms")
```
Here's the optimized code:
```
def fault_classification_wrapper(vin, main_path, data_path, log_path, done_path):
start_time = time.time()
isc_path = Path(done_path) / vin / 'isc_cal_result' / f'{vin}_report.xlsx'
if not isc_path.exists():
print('No isc detection input!')
isc_input = pd.DataFrame()
else:
isc_input = isc_produce_alarm(isc_path, vin)
ica_path = Path(done_path) / vin / 'ica_cal_result' / f'ica_detection_alarm_{vin}.csv'
if not ica_path.exists():
print('No ica detection input!')
ica_input = pd.DataFrame()
else:
ica_input = ica_produce_alarm(ica_path)
soh_path = Path(done_path) / vin / 'SOH_cal_result' / f'{vin}_sohAno.csv'
if not soh_path.exists():
print('No soh detection input!')
soh_input = pd.DataFrame()
else:
soh_input = soh_produce_alarm(soh_path, vin)
alarm_df = pd.concat([isc_input, ica_input, soh_input]).reset_index(drop=True)
alarm_df['alarm_cell'] = alarm_df['alarm_cell'].apply(str)
print(vin)
module = AutoAnalysisMain(alarm_df, main_path, data_path, done_path)
module.analysis_process()
with open(Path(log_path) / 'log.txt', 'w') as txt_file:
for k, v in module.output.items():
txt_file.write(f"{k}:{v}\n")
for x, y in module.output_sub.items():
txt_file.write(f"{x}:{y}\n\n")
fc_result_path = Path(done_path) / vin / 'fc_result'
fc_result_path.mkdir(parents=True, exist_ok=True)
pd.DataFrame(module.output).to_csv(fc_result_path / 'main_structure.csv')
pd.DataFrame(module.output_sub).to_csv(fc_result_path / 'sub_structure.csv')
end_time = time.time()
print(f"time cost of fault classification: {(end_time - start_time) * 1000.0} ms")
return
```
相关推荐
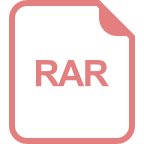













