public void run() { long start = System.currentTimeMillis(); while (this.v > 0) { if (this.v > 0 && this.flow > 0) { this.v = this.v - this.flow; } System.out.println("线程" + Thread.currentThread().getName() + "剩余" + v); } long end = System.currentTimeMillis();
时间: 2023-06-11 08:09:50 浏览: 47
) { System.out.println("Thread " + Thread.currentThread().getName() + " is running: " + this.v); this.v--; } try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } long end = System.currentTimeMillis(); System.out.println("Thread " + Thread.currentThread().getName() + " finished in " + (end - start) / 1000 + " seconds."); }
这段代码是一个线程的run()方法实现,它会不断地打印当前的计数器值,并每隔1秒钟将计数器减1,直到计数器为0。在线程执行完毕后,会记录线程的执行时间并输出。这段代码可以用于实现倒计时的功能,或者是类似任务执行的进度条等场景。
相关问题
解释一下这段Java代码 public boolean time(int time) { final boolean[] Flag = {false}; new Thread(new Runnable() { @Override public void run() { long currentTime = System.currentTimeMillis(); long timeCurrent = 0; while (true) { if(timeCurrent > time) { Flag[0] = true; break; } timeCurrent = System.currentTimeMillis() - currentTime; } } }).start(); return Flag[0]; }
这段代码定义了一个名为`time`的方法,该方法接受一个整数参数`time`,并返回一个布尔值。
在方法内部,首先创建了一个`Flag`数组,数组中只有一个元素,初始值为`false`。接下来,通过创建一个新的线程来执行一个匿名的`Runnable`对象。
在这个匿名的`Runnable`对象的`run`方法中,首先获取当前时间戳`currentTime`。然后,进入一个无限循环。在每次循环中,通过计算当前时间戳与初始时间戳的差值,得到经过的时间`timeCurrent`。
然后,通过比较`timeCurrent`与传入的参数`time`的大小关系,如果超过了指定的时间,将数组中的元素`Flag[0]`设置为`true`,并使用`break`语句跳出循环。
最后,在新线程启动后,立即返回数组元素`Flag[0]`的值。请注意,由于新线程是在后台执行的,因此无法保证在调用方法后立即获得最终结果。可能需要等待一段时间,直到新线程完成循环并设置了正确的布尔值。
public class Main { public static boolean isPrime(int n) { if(n < 2) return false; if(n == 2) return true; if(n%2==0) return false; for(int i = 3; i < n; i += 2) if(n%i == 0) return false; return true; } public static void main(String[] args) throws InterruptedException { long time1 = System.currentTimeMillis(),time2; Task task1 = new Task(1,100000); Task task2 = new Task(100001,200000); Thread thread1 = new Thread(task1); Thread thread2 = new Thread(task2); thread1.start(); thread2.start(); while (thread1.isAlive() || thread2.isAlive()){ Thread.sleep(1); } time2 = System.currentTimeMillis(); System.out.println("总耗时:"+(time2 - time1)+"毫秒"); } } class Task implements Runnable{ private int start; private int end; Task(int start, int end) { this.start = start; this.end = end; } public void run() { long time = System.currentTimeMillis(); long count = 0; for(int i=start;i<=end;++i){ if(Main.isPrime(i)) count+=i; } System.out.println(String.format("%d-%d之间质数和为%d,耗时:%d毫秒",start,end,count,(System.currentTimeMillis()- time))); } }每句加注释
这是一个Java程序,包含一个Main类和一个Task类,代码如下:
```java
public class Main {
// 判断一个数是否为质数
public static boolean isPrime(int n) {
if (n < 2) return false;
if (n == 2) return true;
if (n % 2 == 0) return false;
for (int i = 3; i < n; i += 2)
if (n % i == 0) return false;
return true;
}
public static void main(String[] args) throws InterruptedException {
long time1 = System.currentTimeMillis(), time2;
// 创建两个任务,分别计算1-100000和100001-200000之间的质数和
Task task1 = new Task(1, 100000);
Task task2 = new Task(100001, 200000);
// 创建两个线程,分别执行两个任务
Thread thread1 = new Thread(task1);
Thread thread2 = new Thread(task2);
// 启动线程
thread1.start();
thread2.start();
// 等待两个线程执行完毕
while (thread1.isAlive() || thread2.isAlive()) {
Thread.sleep(1);
}
time2 = System.currentTimeMillis();
System.out.println("总耗时:" + (time2 - time1) + "毫秒");
}
}
class Task implements Runnable {
private int start; // 起始数值
private int end; // 结束数值
Task(int start, int end) {
this.start = start;
this.end = end;
}
public void run() {
long time = System.currentTimeMillis();
long count = 0;
// 计算起始数值到结束数值之间的质数和
for (int i = start; i <= end; ++i) {
if (Main.isPrime(i)) count += i;
}
// 输出计算结果和耗时
System.out.println(String.format("%d-%d之间质数和为%d,耗时:%d毫秒", start, end, count, (System.currentTimeMillis() - time)));
}
}
```
其中,Main类的`isPrime`方法用于判断一个数是否为质数,采用了常见的质数判定算法;`main`方法创建了两个任务,分别计算1-100000和100001-200000之间的质数和,创建了两个线程分别执行这两个任务,等待线程执行完毕后输出总耗时;Task类实现了Runnable接口,用于执行具体的任务,其中的`run`方法计算起始数值到结束数值之间的质数和,输出计算结果和耗时。
相关推荐
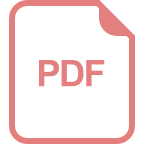
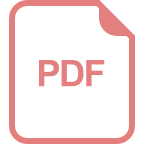












