用easyx画五角星
时间: 2024-12-25 16:21:22 浏览: 6
### 如何使用EasyX图形库绘制五角星
为了实现五角星的绘制,可以利用EasyX图形库提供的绘图功能。下面是一个完整的C++程序示例,展示了如何通过计算五个顶点的位置来画出一个正五角星。
```cpp
#include <graphics.h>
#include <math.h>
void DrawFivePointedStar(int centerX, int centerY, int outerRadius) {
const double PI = 3.1415926;
const int numPoints = 5; // Five points of the star
POINT points[numPoints * 2]; // For alternating inner and outer points
for (int i = 0; i < numPoints; ++i) {
// Calculate angle between each point
float theta = ((float)i / numPoints + 0.5f) * 2.0f * PI;
// Outer vertex calculation
points[i * 2].x = static_cast<int>(centerX + cos(theta) * outerRadius);
points[i * 2].y = static_cast<int>(centerY - sin(theta) * outerRadius);
// Inner vertices are calculated by shifting index forward one position with modulo operation.
int nextIndex = (i + 2) % numPoints;
float innerTheta = ((float)nextIndex / numPoints + 0.5f) * 2.0f * PI;
// Assuming an approximate ratio for simplicity here as half radius for inner circle
float innerRadius = outerRadius / 2.7f;
points[(i * 2) + 1].x = static_cast<int>(centerX + cos(innerTheta) * innerRadius);
points[(i * 2) + 1].y = static_cast<int>(centerY - sin(innerTheta) * innerRadius);
}
setlinecolor(RGB(255, 0, 0)); // Set line color to red
polygon(points, sizeof(points)/sizeof(points[0])); // Draw filled or outlined based on your need
}
int main() {
initgraph(800, 600); // Initialize graphic window size
DrawFivePointedStar(400, 300, 150); // Call function to draw a five-pointed star at center location
getchar(); // Wait until user presses any key before closing the graph window
closegraph();
}
```
这段代码定义了一个`DrawFivePointedStar()`函数用于创建并连接构成五角星形状的一系列坐标点,并调用了EasyX库中的`polygon()`方法完成实际的绘画工作[^1]。
阅读全文
相关推荐
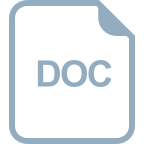
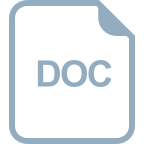
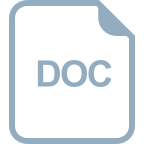

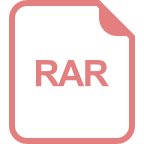












