NX 二次开发 获取一个面上的最长边代码实现
时间: 2023-11-27 13:54:56 浏览: 30
NX 是一个Python库,可以用于网络分析。如果要获取一个面上的最长边,可以按照以下步骤:
1. 导入必要的库
```python
import networkx as nx
from shapely.geometry import LineString
```
2. 创建一个空的无向图
```python
G = nx.Graph()
```
3. 添加节点和边
假设有一个面的坐标列表为coords,则可以使用以下代码将每个坐标添加为图的一个节点,并将相邻的节点之间添加为一条边:
```python
for i, coord in enumerate(coords):
G.add_node(i, pos=coord)
if i > 0:
G.add_edge(i-1, i)
if i == len(coords)-1:
G.add_edge(i, 0)
```
4. 计算每条边的长度
```python
edge_lengths = {}
for i, j in G.edges():
x1, y1 = G.nodes[i]['pos']
x2, y2 = G.nodes[j]['pos']
length = LineString([(x1,y1), (x2,y2)]).length
edge_lengths[(i,j)] = length
```
5. 找到最长的边
```python
longest_edge = max(edge_lengths, key=edge_lengths.get)
```
完整代码示例:
```python
import networkx as nx
from shapely.geometry import LineString
# 坐标列表
coords = [(0,0), (1,1), (2,3), (4,7), (6,8), (8,5), (7,3), (5,1), (3,0)]
# 创建空图
G = nx.Graph()
# 添加节点和边
for i, coord in enumerate(coords):
G.add_node(i, pos=coord)
if i > 0:
G.add_edge(i-1, i)
if i == len(coords)-1:
G.add_edge(i, 0)
# 计算每条边的长度
edge_lengths = {}
for i, j in G.edges():
x1, y1 = G.nodes[i]['pos']
x2, y2 = G.nodes[j]['pos']
length = LineString([(x1,y1), (x2,y2)]).length
edge_lengths[(i,j)] = length
# 找到最长的边
longest_edge = max(edge_lengths, key=edge_lengths.get)
print(f"The longest edge is between nodes {longest_edge[0]} and {longest_edge[1]}, with a length of {edge_lengths[longest_edge]}")
```
相关推荐
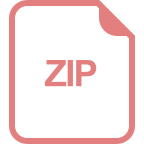
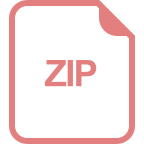














