如何让Mean Shift自适应带宽的代码实现
时间: 2024-03-10 15:51:25 浏览: 20
以下是使用 Python 实现自适应带宽 Mean Shift 算法的示例代码:
```python
import numpy as np
def adaptive_bandwidth(X, c):
"""
计算自适应带宽
:param X: 样本数据,numpy array,shape为(n_samples, n_features)
:param c: 常数,用于调整带宽大小
:return: 自适应带宽,numpy array,shape为(n_samples,)
"""
n_samples, n_features = X.shape
# 计算每个样本点之间的欧式距离矩阵
dist_matrix = np.zeros((n_samples, n_samples))
for i in range(n_samples):
for j in range(i+1, n_samples):
dist_matrix[i][j] = np.sqrt(np.sum((X[i]-X[j])**2))
dist_matrix[j][i] = dist_matrix[i][j]
# 计算每个样本点的密度
density = np.zeros(n_samples)
for i in range(n_samples):
density[i] = np.sum(np.exp(-dist_matrix[i]**2/2))
# 计算每个样本点的自适应带宽
bandwidth = np.zeros(n_samples)
for i in range(n_samples):
bandwidth[i] = c / np.sqrt(density[i])
return bandwidth
def mean_shift(X, bandwidth, threshold):
"""
计算均值漂移后的样本点
:param X: 样本数据,numpy array,shape为(n_samples, n_features)
:param bandwidth: 带宽,numpy array,shape为(n_samples,)
:param threshold: 阈值,用于判断两个样本点是否属于同一类
:return: 漂移后的样本点,numpy array,shape为(n_samples, n_features)
"""
n_samples, n_features = X.shape
# 迭代计算每个样本点的均值漂移
for i in range(n_samples):
x = X[i]
b = bandwidth[i]
while True:
# 计算每个样本点与当前点的距离
dist = np.sqrt(np.sum((X-x)**2, axis=1))
# 计算每个样本点的权重
weight = np.exp(-dist**2/(2*b**2))
# 计算每个样本点的均值
mean = np.sum(X*weight.reshape(-1, 1), axis=0) / np.sum(weight)
# 判断均值是否收敛
if np.sum((mean-x)**2) < threshold:
X[i] = mean
break
x = mean
return X
def cluster(X, bandwidth, threshold):
"""
对均值漂移后的样本点进行聚类
:param X: 均值漂移后的样本点,numpy array,shape为(n_samples, n_features)
:param bandwidth: 带宽,numpy array,shape为(n_samples,)
:param threshold: 阈值,用于判断两个样本点是否属于同一类
:return: 聚类结果,list,每个元素为一个类别,其中每个元素为一个样本点的索引
"""
n_samples, n_features = X.shape
visited = np.zeros(n_samples)
clusters = []
# 对每个样本点进行聚类
for i in range(n_samples):
if visited[i] == 0:
cluster_i = [i]
visited[i] = 1
for j in range(i+1, n_samples):
if visited[j] == 0 and np.sum((X[i]-X[j])**2 / bandwidth[i]**2) < threshold:
cluster_i.append(j)
visited[j] = 1
clusters.append(cluster_i)
return clusters
# 示例代码
X = np.random.rand(100, 2)
bandwidth = adaptive_bandwidth(X, 1)
X_shifted = mean_shift(X, bandwidth, 1e-5)
clusters = cluster(X_shifted, bandwidth, 0.1)
print(clusters)
```
上述代码中,`adaptive_bandwidth` 函数用于计算自适应带宽,`mean_shift` 函数用于计算均值漂移后的样本点,`cluster` 函数用于对均值漂移后的样本点进行聚类。
需要注意的是,上述代码中的阈值参数 `threshold` 可以根据具体的数据集和应用场景进行调整。
相关推荐
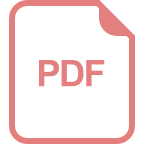














