使用JAVA写一个快速排序
时间: 2023-08-31 22:29:25 浏览: 93
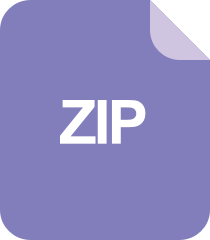
基于 Java 实现的快速排序算法(java 实现方式)
### 回答1:
我可以提供一个简单的Java快速排序示例:
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (arr == null || arr.length == 0)
return;
if (low >= high)
return;
// pick the pivot
int middle = low + (high - low) / 2;
int pivot = arr[middle];
// make left < pivot and right > pivot
int i = low, j = high;
while (i <= j) {
while (arr[i] < pivot) {
i++;
}
while (arr[j] > pivot) {
j--;
}
if (i <= j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
i++;
j--;
}
}
// recursively sort two sub parts
if (low < j)
quickSort(arr, low, j);
if (high > i)
quickSort(arr, i, high);
}
}
### 回答2:
快速排序是一种常用的排序算法,也是一种高效的排序算法。下面是使用Java语言实现的快速排序算法:
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (arr == null || arr.length == 0 || low >= high) {
return;
}
int left = low;
int right = high;
int pivot = arr[low + (high - low) / 2]; // 选择中间元素作为枢纽元
while (left <= right) {
while (arr[left] < pivot) {
left++;
}
while (arr[right] > pivot) {
right--;
}
if (left <= right) {
swap(arr, left, right);
left++;
right--;
}
}
if (low < right) {
quickSort(arr, low, right);
}
if (high > left) {
quickSort(arr, left, high);
}
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {5, 9, 1, 3, 7, 6};
quickSort(arr, 0, arr.length - 1);
System.out.println("排序后的数组:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
在快速排序实现中,首先选择枢纽元素(一般选择待排序数组的中间元素)作为基准,然后根据基准元素对数组进行划分,使得左边的元素都小于基准值,右边的元素都大于基准值。然后递归地对左右两个区间进行快速排序。最终,当子数组的长度不再大于1时,排序完成。
以上为快速排序的Java实现,通过递归实现了对给定整型数组的快速排序,最终打印排序后的数组结果。
### 回答3:
快速排序是一种经典的排序算法,使用递归来对一个数组进行排序。下面是使用JAVA编写的快速排序算法:
```java
public class QuickSort {
public static void quickSort(int[] arr, int left, int right) {
if (left < right) {
int partitionIndex = partition(arr, left, right);
quickSort(arr, left, partitionIndex-1);
quickSort(arr, partitionIndex+1, right);
}
}
public static int partition(int[] arr, int left, int right) {
int pivot = arr[right];
int i = (left-1);
for (int j = left; j < right; j++) {
if (arr[j] < pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i+1, right);
return i+1;
}
public static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {10, 7, 8, 9, 1, 5};
int n = arr.length;
quickSort(arr, 0, n-1);
System.out.println("排序后的数组:");
for (int i = 0; i < n; ++i) {
System.out.print(arr[i] + " ");
}
}
}
```
上面的代码首先定义了一个`quickSort`函数来实现快速排序。在这个函数中,我们首先选择一个基准元素(一般取最后一个元素),并通过调用`partition`函数将数组分成两个部分。`partition`函数使用两个指针来将小于基准元素的元素放到基准元素的左边,大于基准元素的元素放到基准元素的右边,最终返回基准元素的索引。然后我们递归地调用`quickSort`函数来对基准元素的左边和右边的子数组进行排序。
在`partition`函数中,我们首先将基准元素设置为数组的最后一个元素,并定义一个变量`i`来记录小于基准元素的元素的最后一个位置。然后我们遍历整个数组,如果当前元素小于基准元素,就将它与`i`指向的元素交换,并将`i`向后移动一位。最后我们将基准元素与`i+1`位置的元素交换,返回`i+1`作为新的基准元素的索引。
最后,在`main`函数中我们定义一个测试数组,并调用`quickSort`函数来对数组进行排序。排序完成后,我们将数组打印出来以验证排序结果。
阅读全文
相关推荐
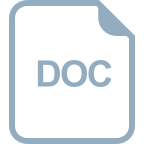
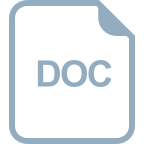
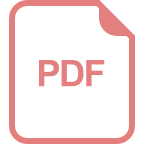
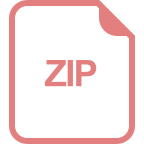
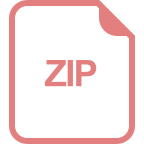
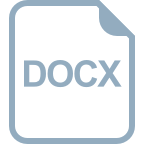
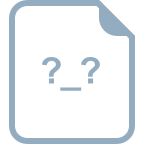
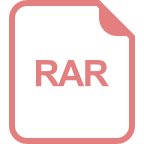
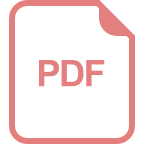
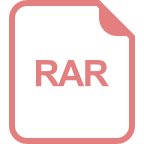
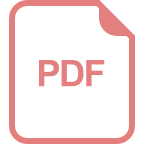
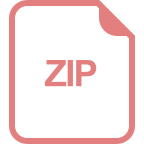
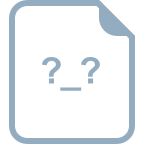
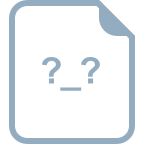