用顺序表表示整数集合 A, B,实现 A = A ∪ B 和A = A ∩ B。
时间: 2024-11-03 08:17:09 浏览: 23
使用顺序表(数组)表示整数集合A和B并实现集合的并集(A ∪ B)和交集(A ∩ B),我们需要考虑以下步骤:
### 集合操作基本概念
- **并集** (∪): 包含两个集合中的所有元素,无重复。
- **交集** (∩): 只包含同时存在于两个集合中的元素。
### 实现思路
1. **顺序表**: 我们通常会创建两个指针,一个指向A的第一个元素,另一个指向B的第一个元素。遍历两个列表,如果某个元素不在当前列表中,则添加到结果集中。
2. **A ∪ B**: 遍历B的同时检查每个元素是否已经在A中。如果没有,在新列表中添加。
3. **A ∩ B**: 类似地,但只有当找到的元素既在A也在B时才添加到结果中。
### C语言代码示例
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int* elements;
size_t size;
} SequenceList;
void add_element(SequenceList* list, int element) {
if (list->size == list->elements capacity) {
// 扩展序列列表容量
list->elements = realloc(list->elements, (list->size + 1) * sizeof(int));
}
list->elements[list->size++] = element;
}
// 判断元素是否已经存在于列表中
int is_in_list(const SequenceList* list, int element) {
for (size_t i = 0; i < list->size; ++i) {
if (list->elements[i] == element)
return 1;
}
return 0;
}
void union_set(SequenceList* a, SequenceList* b) {
for (int i = 0; i < b->size; ++i) {
if (!is_in_list(a, b->elements[i])) {
add_element(a, b->elements[i]);
}
}
}
void intersection_set(SequenceList* a, SequenceList* b) {
SequenceList temp;
temp.elements = malloc(sizeof(int)); // 创建临时列表
temp.size = 0;
for (int i = 0; i < a->size; ++i) {
if (is_in_list(b, a->elements[i])) { // 如果a的元素在b中
add_element(&temp, a->elements[i]); // 添加到临时列表
}
}
free(a->elements); // 释放原A的内存
a->elements = temp.elements;
a->size = temp.size;
}
void print_sequence_list(SequenceList* list) {
printf("[");
for (size_t i = 0; i < list->size; ++i) {
printf("%d", list->elements[i]);
if (i != list->size - 1)
printf(", ");
}
printf("]\n");
}
int main() {
SequenceList a = {NULL, 0};
SequenceList b = {NULL, 0};
// 初始化你的A和B列表...
union_set(&a, &b);
printf("Union of A and B: ");
print_sequence_list(&a);
intersection_set(&a, &b);
printf("\nIntersection of A and B: ");
print_sequence_list(&a);
return 0;
}
```
阅读全文
相关推荐
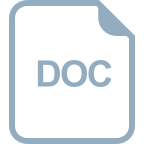
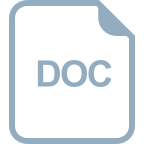
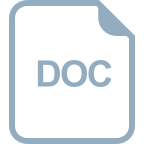
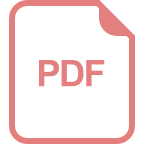
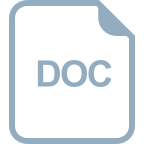
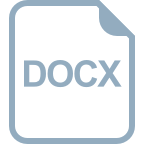
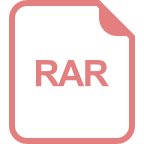
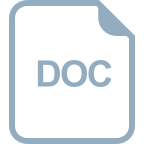
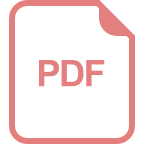
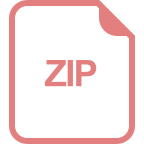
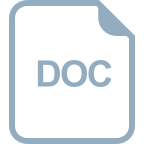
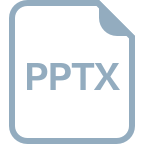
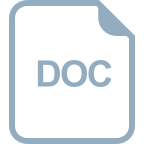
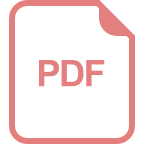
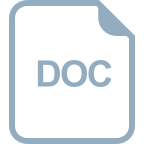
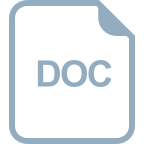
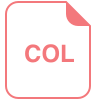
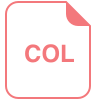
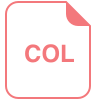