目标网页:https://www.lagou.com/zhaopin/ 完成重庆市计算机行业工作的查找,月薪5k-10k 要求调用到的方法: 1.下拉菜单的选择; 2.xpath定位
时间: 2023-11-30 22:04:37 浏览: 77
以下是使用 Python 和 Selenium 实现的代码:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import Select
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# 创建浏览器对象,需提前安装 Chrome 浏览器和对应的 ChromeDriver
driver = webdriver.Chrome()
# 打开目标网页
driver.get("https://www.lagou.com/zhaopin/")
# 等待页面加载完成
wait = WebDriverWait(driver, 10)
wait.until(EC.presence_of_element_located((By.XPATH, "//div[@class='body-container']")))
# 选择城市为重庆
city_select = driver.find_element_by_xpath("//div[@data-property='city']")
city_select.click()
city_options = Select(city_select.find_element_by_tag_name('select'))
city_options.select_by_visible_text('重庆')
# 选择计算机软件行业
industry_select = driver.find_element_by_xpath("//div[@data-property='industry']")
industry_select.click()
industry_options = Select(industry_select.find_element_by_tag_name('select'))
industry_options.select_by_visible_text('计算机软件')
# 输入搜索关键字并提交
search_input = driver.find_element_by_xpath("//input[@placeholder='搜索职位、公司或地点']")
search_input.send_keys("工作")
search_input.send_keys(Keys.RETURN)
# 选择月薪5k-10k
salary_select = driver.find_element_by_xpath("//li[@data-option='5k-10k']")
salary_select.click()
# 等待搜索结果加载完成
wait.until(EC.presence_of_element_located((By.XPATH, "//div[@class='s_position_list ']")))
# 输出搜索结果
job_list = driver.find_elements_by_xpath("//li[@class='con_list_item']")
for job in job_list:
print(job.text)
# 关闭浏览器
driver.quit()
```
注意,此代码仅供学习参考,具体的网页元素定位可能会因为网页结构的变化而失效。
阅读全文
相关推荐
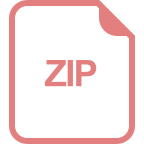
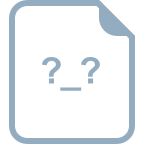















