使用Levenberg-Marquardt算法对相机标定结果进行优化的代码(matlab)
时间: 2023-07-01 08:08:21 浏览: 123
以下是使用Levenberg-Marquardt算法对相机标定结果进行优化的matlab代码示例:
```matlab
% 假设已经完成相机标定并获得了初始的内参和外参矩阵
% K为内参矩阵,R、T为外参矩阵
% 定义优化所需的变量
x0 = [K(1,1), K(2,2), K(1,3), K(2,3), R(1,1), R(1,2), R(1,3), R(2,1), R(2,2), R(2,3), R(3,1), R(3,2), R(3,3), T(1), T(2), T(3)];
% 定义观测数据,包括标定板上每个角点的二维坐标和对应的三维空间坐标
observed_points = [image_points; world_points];
% 定义误差函数
fun = @(x) calibrate_error(x, observed_points, image_size);
% 使用Levenberg-Marquardt算法进行优化
options = optimoptions('lsqnonlin','Algorithm','levenberg-marquardt');
[x,resnorm] = lsqnonlin(fun,x0,[],[],options);
% 将优化结果转换为内参和外参矩阵
K_optimized = [x(1), 0, x(3); 0, x(2), x(4); 0, 0, 1];
R_optimized = [x(5), x(6), x(7); x(8), x(9), x(10); x(11), x(12), x(13)];
T_optimized = [x(14); x(15); x(16)];
```
其中,calibrate_error函数用于计算标定误差,具体实现可以参考以下示例代码:
```matlab
function error = calibrate_error(x, data, image_size)
% 计算标定误差
K = [x(1), 0, x(3); 0, x(2), x(4); 0, 0, 1];
R = [x(5), x(6), x(7); x(8), x(9), x(10); x(11), x(12), x(13)];
T = [x(14); x(15); x(16)];
num_points = size(data, 1) / 2;
observed_points = data(1:num_points, :);
world_points = data(num_points+1:end, :);
% 将观测点从像素坐标系转换到摄像机坐标系
camera_points = observed_points - repmat([K(1,3), K(2,3)], num_points, 1);
camera_points(:,1) = camera_points(:,1) / K(1,1);
camera_points(:,2) = camera_points(:,2) / K(2,2);
% 将世界坐标系下的点转换到相机坐标系下
camera_world_points = (R * world_points')' + repmat(T', num_points, 1);
% 将相机坐标系下的点转换到像素坐标系下
projected_points = camera_world_points(:,1:2) ./ repmat(camera_world_points(:,3), 1, 2);
projected_points(:,1) = projected_points(:,1) * K(1,1) + K(1,3);
projected_points(:,2) = projected_points(:,2) * K(2,2) + K(2,3);
% 计算误差
error = [projected_points(:,1) - observed_points(:,1); projected_points(:,2) - observed_points(:,2)];
error = error(:);
end
```
其中,data参数为观测数据,image_size参数为图像尺寸。在计算误差时,首先将观测点从像素坐标系转换到摄像机坐标系,然后将世界坐标系下的点转换到相机坐标系下,最后将相机坐标系下的点转换到像素坐标系下,并计算预测点与观测点之间的误差。
阅读全文
相关推荐
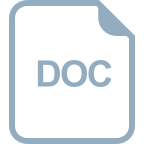
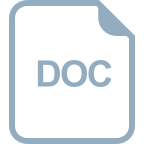
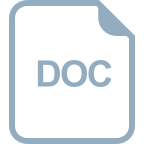






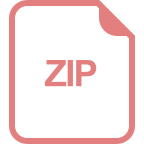
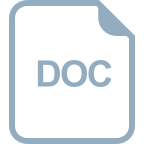
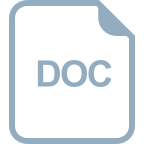
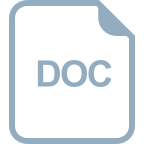



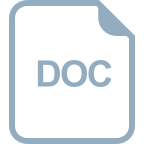