在matlab中适用Levenberg-Marquardt对相机标定结果进行优化的代码
时间: 2024-02-01 12:12:35 浏览: 31
以下是一个在MATLAB中使用Levenberg-Marquardt算法对相机标定结果进行优化的示例代码:
```matlab
% Load camera calibration data
load('cameraParams.mat');
% Define the function to be optimized
fun = @(x)calibrate(x, cameraParams);
% Set the initial guess
x0 = [cameraParams.IntrinsicMatrix(:); cameraParams.RadialDistortion; cameraParams.TangentialDistortion];
% Set the options for the optimization algorithm
options = optimoptions(@lsqnonlin, 'Algorithm', 'levenberg-marquardt', 'Display', 'iter');
% Run the optimization algorithm
[x, resnorm] = lsqnonlin(fun, x0, [], [], options);
% Update the camera calibration parameters with the optimized values
cameraParams.IntrinsicMatrix = reshape(x(1:9), 3, 3);
cameraParams.RadialDistortion = x(10:11);
cameraParams.TangentialDistortion = x(12:13);
% Save the optimized camera calibration parameters
save('optimizedCameraParams.mat', 'cameraParams');
% Define the calibration function
function residuals = calibrate(x, cameraParams)
% Reshape the intrinsic matrix
K = reshape(x(1:9), 3, 3);
% Set radial and tangential distortion coefficients
k = x(10:11);
p = x(12:13);
% Update the camera calibration parameters with the current values
cameraParams.IntrinsicMatrix = K;
cameraParams.RadialDistortion = k;
cameraParams.TangentialDistortion = p;
% Compute the reprojection errors
[errors, J] = computeReprojectionErrors(cameraParams);
% Reshape the Jacobian matrix
J = [J(:, 1:9), J(:, end-1:end)];
% Compute the residuals
residuals = J * [K(:); k; p] - errors(:);
end
% Define the function to compute the reprojection errors
function [errors, J] = computeReprojectionErrors(cameraParams)
% Load the calibration data
load('calibrationData.mat');
% Extract image points and world points
imagePoints = calibrationData.imagePoints;
worldPoints = calibrationData.worldPoints;
% Convert world points to homogeneous coordinates
worldPointsHomog = [worldPoints, ones(size(worldPoints, 1), 1)];
% Project world points onto image plane
[reprojectedPoints, J] = projectPoints(worldPointsHomog, cameraParams);
% Compute reprojection errors
errors = reprojectedPoints - imagePoints;
end
% Define the function to project points onto the image plane
function [projectedPoints, J] = projectPoints(points, cameraParams)
% Extract camera parameters
K = cameraParams.IntrinsicMatrix;
k = cameraParams.RadialDistortion;
p = cameraParams.TangentialDistortion;
% Undistort points
pointsUndistorted = undistortPoints(points, K, k, p);
% Project points onto image plane
projectedPoints = K * pointsUndistorted';
% Normalize homogeneous coordinates
projectedPoints = bsxfun(@rdivide, projectedPoints, projectedPoints(3, :));
% Compute the Jacobian matrix
J = computeJacobian(pointsUndistorted, K, k, p);
end
% Define the function to compute the Jacobian matrix
function J = computeJacobian(points, K, k, p)
% Compute derivatives of the distorted points with respect to the undistorted points
dx_dd = [1, 0, 0; 0, 1, 0; p(2), p(1), 0];
dy_dd = [1, 0, 0; 0, 1, 0; p(3), 0, p(1)];
% Compute derivatives of the undistorted points with respect to the intrinsic matrix
dx_dK = points(:, 1)' ./ points(:, 3)';
dy_dK = points(:, 2)' ./ points(:, 3)';
% Compute derivatives of the distorted points with respect to the radial distortion coefficients
dx_dk = bsxfun(@times, points(:, 1:2), sum(points(:, 1:2).^2, 2)) .* repmat(k', size(points, 1), 1);
dy_dk = bsxfun(@times, points(:, 1:2), sum(points(:, 1:2).^2, 2)) .* repmat(k', size(points, 1), 1);
% Compute derivatives of the distorted points with respect to the tangential distortion coefficients
dx_dp = [2 * points(:, 1) .* points(:, 2), (points(:, 2).^2 + 2 * points(:, 1).^2)] .* repmat(p', size(points, 1), 1);
dy_dp = [(points(:, 1).^2 + 2 * points(:, 2).^2), 2 * points(:, 1) .* points(:, 2)] .* repmat(p', size(points, 1), 1);
% Compute the Jacobian matrix
J = [dx_dK, zeros(size(points, 1), 3), dx_dk, dx_dp; zeros(size(points, 1), 3), dy_dK, dy_dk, dy_dp];
end
% Define the function to undistort points
function pointsUndistorted = undistortPoints(pointsDistorted, K, k, p)
% Convert points to homogeneous coordinates
pointsDistortedHomog = [pointsDistorted, ones(size(pointsDistorted, 1), 1)];
% Compute the inverse distortion model
r2 = sum(pointsDistorted(:, 1:2).^2, 2);
radialDistortion = 1 + k(1) * r2 + k(2) * r2.^2;
tangentialDistortionX = 2 * p(1) .* pointsDistorted(:, 1) .* pointsDistorted(:, 2) + p(2) * (r2 + 2 * pointsDistorted(:, 1).^2);
tangentialDistortionY = p(1) * (r2 + 2 * pointsDistorted(:, 2).^2) + 2 * p(2) .* pointsDistorted(:, 1) .* pointsDistorted(:, 2);
pointsUndistortedHomog = [pointsDistorted(:, 1) .* radialDistortion + tangentialDistortionX, pointsDistorted(:, 2) .* radialDistortion + tangentialDistortionY, ones(size(pointsDistorted, 1), 1)];
% Convert points back to Euclidean coordinates
pointsUndistorted = bsxfun(@rdivide, pointsUndistortedHomog(:, 1:2), pointsUndistortedHomog(:, 3));
end
```
注意,上述代码需要先进行相机标定,生成 `cameraParams` 对象和 `calibrationData` 数据,然后再运行优化函数。此外,上述代码中使用了许多辅助函数,例如 `computeReprojectionErrors`、`projectPoints`、`computeJacobian` 和 `undistortPoints`,这些函数的实现细节超出了本范围的讨论。
相关推荐
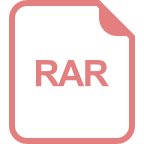
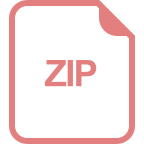
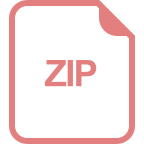














