我现在在unity中用using UnityEngine; using UnityEditor; public class CreateAsset : EditorWindow { [MenuItem("Assets/Create/My Asset")] public static void CreateMyAsset() { MyAsset asset = ScriptableObject.CreateInstance<MyAsset>(); AssetDatabase.CreateAsset(asset, "Assets/NewMyAsset.asset"); AssetDatabase.SaveAssets(); EditorUtility.FocusProjectWindow(); Selection.activeObject = asset; } } public class MyAsset : ScriptableObject { public int myInt; public string myString; }这种方式生成了一个.asset文件,现在写一个脚本,脚本里有一个public变量,把生成的.asset文件拖拽到这个变量上
时间: 2024-03-10 09:48:46 浏览: 22
好的,你可以在你的脚本中声明一个public变量,类型为MyAsset类。然后在Unity编辑器中,将你生成的MyAsset类型的.asset文件拖拽到这个变量上。以下是一个示例:
```csharp
using UnityEngine;
public class MyScript : MonoBehaviour
{
// 声明一个public变量,类型为MyAsset类
public MyAsset myAsset;
void Start ()
{
// 使用myAsset变量
Debug.Log("MyAsset myInt: " + myAsset.myInt);
Debug.Log("MyAsset myString: " + myAsset.myString);
}
}
```
在上面的示例中,我们声明了一个public变量myAsset,类型为MyAsset类。我们可以将生成的MyAsset类型的.asset文件拖拽到该变量上,从而将该文件赋值给myAsset变量。在Start()函数中,我们使用了myAsset变量,并输出了该变量的myInt和myString字段的值。
相关问题
using UnityEngine; using UnityEditor; public class CreateAsset : EditorWindow { [MenuItem("Assets/Create/My Asset")] public static void CreateMyAsset() { MyAsset asset = ScriptableObject.CreateInstance<MyAsset>(); AssetDatabase.CreateAsset(asset, "Assets/NewMyAsset.asset"); AssetDatabase.SaveAssets(); EditorUtility.FocusProjectWindow(); Selection.activeObject = asset; } } public class MyAsset : ScriptableObject { public int myInt; public string myString; },这个代码生成的.asset文件如何读取?
要读取.asset文件,您需要使用Unity中的AssetDatabase类和AssetDatabase.LoadAssetAtPath方法。这里是一个简单的示例代码,演示如何加载并打印MyAsset.asset文件中的myInt和myString属性:
```
using UnityEngine;
using UnityEditor;
public class ReadAsset : EditorWindow
{
[MenuItem("Assets/Read My Asset")]
public static void ReadMyAsset()
{
MyAsset asset = AssetDatabase.LoadAssetAtPath<MyAsset>("Assets/NewMyAsset.asset");
if(asset != null)
{
Debug.Log("MyInt: " + asset.myInt);
Debug.Log("MyString: " + asset.myString);
}
else
{
Debug.LogError("Failed to load MyAsset asset.");
}
}
}
```
在Unity Editor中,您可以通过单击“Assets”窗口中的NewMyAsset.asset文件,然后单击菜单栏中的“Read My Asset”选项来运行此代码。该代码将加载该文件并输出myInt和myString属性的值。
unity 内制作.asset文件
在Unity中,你可以通过AssetDatabase类来创建.asset文件。AssetDatabase是Unity编辑器中一个非常重要的类,它提供了许多与Asset相关的方法,包括创建、删除、移动、复制等等。
下面是一个示例代码,可以在Unity编辑器中创建一个.asset文件:
```csharp
using UnityEngine;
using UnityEditor;
public class CreateAsset : EditorWindow
{
[MenuItem("Assets/Create/My Asset")]
public static void CreateMyAsset()
{
MyAsset asset = ScriptableObject.CreateInstance<MyAsset>();
AssetDatabase.CreateAsset(asset, "Assets/NewMyAsset.asset");
AssetDatabase.SaveAssets();
EditorUtility.FocusProjectWindow();
Selection.activeObject = asset;
}
}
public class MyAsset : ScriptableObject
{
public int myInt;
public string myString;
}
```
上面的代码中,我们定义了一个CreateAsset类,它继承自EditorWindow。CreateMyAsset方法使用AssetDatabase.CreateAsset方法来创建一个新的MyAsset对象,并将其保存为一个.asset文件。然后,我们使用AssetDatabase.SaveAssets方法来保存这个新的.asset文件。最后,我们使用EditorUtility.FocusProjectWindow和Selection.activeObject来选中新创建的.asset文件。
MyAsset类是一个继承自ScriptableObject的自定义类。在这个类中,我们定义了一些字段,可以在创建新的.asset文件时进行设置。
要使用这个示例代码,你需要将其保存为一个.cs文件,并将其放置在Assets/Editor目录下。然后,在Unity编辑器中选择Assets/Create/My Asset菜单项,就可以创建一个新的My Asset对象,并将其保存为一个.asset文件了。
相关推荐
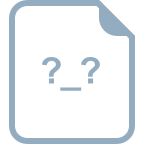
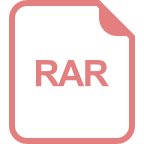
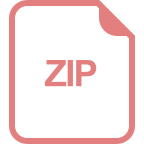










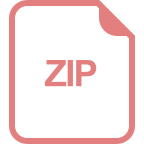
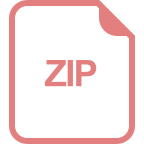
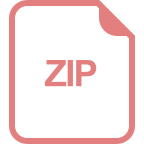