fick diffusion code using random walk method
时间: 2023-12-06 10:44:40 浏览: 22
Sure, I can help you with that. The random walk method is commonly used to simulate diffusion processes in materials. Here's some sample code in Python that implements the random walk method:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define simulation parameters
N = 1000 # Number of particles
steps = 1000 # Number of steps
L = 100 # Size of the system
D = 1 # Diffusion constant
# Initialize particle positions
x = np.zeros(N)
y = np.zeros(N)
# Run simulation
for i in range(steps):
# Update particle positions
x += np.sqrt(2*D)*np.random.randn(N)
y += np.sqrt(2*D)*np.random.randn(N)
# Reflect particles at system boundaries
x = np.mod(x, L)
y = np.mod(y, L)
# Plot particle positions
if i % 10 == 0:
plt.clf()
plt.plot(x, y, 'bo')
plt.xlim([0, L])
plt.ylim([0, L])
plt.draw()
plt.pause(0.0001)
```
In this code, we define the simulation parameters such as the number of particles `N`, the number of steps `steps`, the size of the system `L`, and the diffusion constant `D`. We then initialize the particle positions to be at the origin.
We then loop over the number of steps and update the particle positions using the random walk method. We also reflect the particles at the system boundaries to ensure they stay within the system.
Finally, we plot the particle positions at every 10th step using matplotlib. The `plt.pause(0.0001)` function is used to update the plot in real-time.
Hope this helps! Let me know if you have any questions.
相关推荐
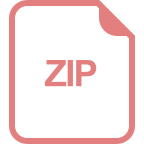
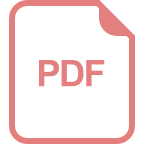














