如何利用LDA模型进行信息检索,用Python 给出例子
时间: 2024-05-06 20:17:55 浏览: 132
LDA (Latent Dirichlet Allocation) 是一种常用的主题模型,它可以通过对文本数据进行分析,发现文本数据中隐含的主题并进行分类。在信息检索中,我们可以使用 LDA 模型来对文本数据进行分类和打上标签,从而实现更高效的检索。
下面是一个使用 Python 进行 LDA 模型信息检索的例子:
1. 首先,需要安装 gensim 和 pyLDAvis 库,可以通过以下命令进行安装:
```python
!pip install gensim pyLDAvis
```
2. 接着,导入所需的库和数据集:
```python
import gensim
import pyLDAvis.gensim_models
from gensim import corpora
from pprint import pprint
# 加载数据集
data = ["This is a sample sentence.",
"Another sample sentence.",
"I love Python programming language.",
"Python is easy to learn and use.",
"Python is widely used in AI and machine learning."]
# 分词和去除停用词
stop_words = set(['is', 'a', 'and', 'the', 'to', 'in', 'of', 'I'])
texts = [[word for word in document.lower().split() if word not in stop_words] for document in data]
```
3. 创建词典和语料库,并训练 LDA 模型:
```python
# 创建词典和语料库
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
# 训练 LDA 模型
lda_model = gensim.models.ldamodel.LdaModel(corpus=corpus, id2word=dictionary, num_topics=3, random_state=100, update_every=1, chunksize=100, passes=10, alpha='auto', per_word_topics=True)
# 打印 LDA 模型的主题
pprint(lda_model.print_topics())
```
4. 可视化 LDA 模型:
```python
# 可视化 LDA 模型
pyLDAvis.enable_notebook()
vis = pyLDAvis.gensim_models.prepare(lda_model, corpus, dictionary)
vis
```
5. 对新文本进行信息检索:
```python
# 对新文本进行信息检索
new_doc = "Python is a popular programming language."
new_doc = [word for word in new_doc.lower().split() if word not in stop_words]
new_doc_bow = dictionary.doc2bow(new_doc)
print(lda_model.get_document_topics(new_doc_bow))
```
以上就是一个简单的使用 LDA 模型进行信息检索的例子。通过对文本数据进行分类和打标签,LDA 模型可以帮助我们更快速、更准确地检索信息。
阅读全文
相关推荐
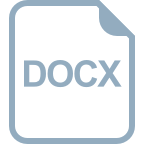
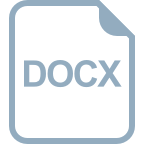
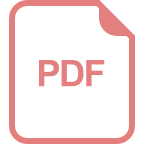















