pytorch里的Linear是什么
时间: 2023-05-25 10:04:15 浏览: 46
在Pytorch中,Linear是一个模块,也叫做全连接层或稠密层。它可以被用来将输入数据映射到输出数据的线性变换。它接受一个输入张量,然后根据权重矩阵和偏置向量进行计算,最终输出一个新的张量,其中输出张量的每个元素是输入张量对应元素的线性组合。在神经网络中,Linear通常被用来表示连接两个相邻的层。
相关问题
pytorch提取linear矩阵权重
### 回答1:
可以使用PyTorch中的`state_dict()`方法来获取模型的参数。具体来说,可以使用以下代码获取`linear`层的权重矩阵:
```
import torch
# 定义模型
class MyModel(torch.nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = torch.nn.Linear(10, 1)
model = MyModel()
# 获取模型参数
params = model.state_dict()
# 获取linear层的权重矩阵
linear_weight = params['linear.weight']
print(linear_weight)
```
这里我们定义了一个简单的模型`MyModel`,包含一个`linear`层。然后使用`state_dict()`方法获取模型参数,最后获取`linear`层的权重矩阵`linear.weight`。
### 回答2:
要提取PyTorch中Linear层的权重矩阵,可以按照以下步骤进行:
首先,导入PyTorch库:
```python
import torch
import torch.nn as nn
```
接下来,定义一个带有Linear层的模型:
```python
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = nn.Linear(in_features=10, out_features=5)
def forward(self, x):
return self.linear(x)
```
对于这个模型,我们可以使用以下代码提取Linear层的参数:
```python
model = MyModel() # 创建模型实例
weights = model.linear.weight # 提取权重矩阵
```
这里,`model.linear`指的是Linear层的实例,通过`.weight`属性可以获取权重矩阵。你可以通过打印输出来查看权重矩阵的值:
```python
print(weights)
```
得到的输出将是一个Tensor对象,包含Linear层的权重矩阵。
需要注意的是,`.weight`属性只能提取Linear层的权重矩阵,而不能提取偏置项。如果你想要同时提取权重矩阵和偏置项,可以使用`.state_dict()`方法。
希望以上回答对你有所帮助。
### 回答3:
在PyTorch中,我们可以通过`state_dict()`方法来提取一个线性层(linear layer)的权重矩阵。`state_dict()`方法返回包含模型参数的字典,其中键是参数的名称,值是对应参数的张量。
我们首先需要定义一个线性层,然后可以使用`state_dict()`方法来提取其权重矩阵。下面是一个示例代码:
```python
import torch
import torch.nn as nn
# 定义一个线性层
linear = nn.Linear(10, 5)
# 提取权重矩阵
weights = linear.state_dict()["weight"]
print(weights)
```
在这个示例中,我们定义了一个大小为(10,5)的线性层。然后,我们通过`state_dict()`方法提取了该线性层的权重矩阵,并将其赋值给变量`weights`。最后,我们打印了权重矩阵。
注意,`state_dict()`方法返回的是一个`OrderedDict`,根据模型中不同层的定义顺序,键的顺序可能会有所不同。你可以根据需要使用键值对来访问模型参数。
pytorch linear
PyTorch Linear is a module in the PyTorch library that implements a linear transformation on a given set of inputs. It is used to build and train neural networks for a wide range of applications such as image classification, natural language processing, and speech recognition.
The PyTorch Linear module is essentially a fully connected layer that takes in a set of inputs and applies a matrix multiplication operation to them along with a bias term. The output of this operation is then passed through an activation function to produce the final output.
The PyTorch Linear module can be initialized with various parameters such as the number of input and output features, the weight initialization method, and the bias initialization method. It can also be used in conjunction with other PyTorch modules such as activation functions, loss functions, and optimizers to build complex neural networks.
Overall, PyTorch Linear is a powerful and flexible tool for building and training neural networks in PyTorch.
相关推荐
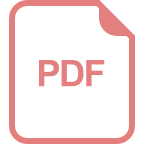
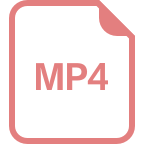
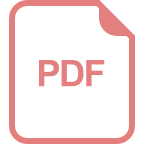












