C++定义一个计算器类,能够实现加减乘除四则运算,对有关成员函数重载,以实现不同类型数据的运算。并用构造函数和析构函数将数据成员初始化为0
时间: 2024-12-02 09:27:48 浏览: 18
在C++中,我们可以定义一个名为Calculator的类来实现一个简单的四则运算计算器,通过成员函数的重载来支持不同类型的运算。以下是这个类的一个基础设计:
```cpp
class Calculator {
private:
double num1; // 第一个操作数
double num2; // 第二个操作数
public:
// 构造函数,用于初始化数据成员
Calculator() : num1(0), num2(0) {}
// 析构函数,清理内存资源
~Calculator() {}
// 加法运算
double add(double another_num = 0) {
return num1 + (another_num ? another_num : num2);
}
// 减法运算
double subtract(double another_num = 0) {
return num1 - (another_num ? another_num : num2);
}
// 乘法运算
double multiply(double another_num = 0) {
return num1 * (another_num ? another_num : num2);
}
// 除法运算,需要处理除数为零的情况
double divide(double another_num = 0) {
if (another_num != 0) {
return num1 / (another_num ? another_num : num2);
} else {
throw std::invalid_argument("Divisor cannot be zero.");
}
}
// 浮点数运算版本,可以重载以接受更广泛的数值类型
template <typename T>
friend T operator+(T a, const Calculator& calc) {
return static_cast<double>(a) + calc.num1;
}
// 其他运算符重载类似
};
```
在这个例子中,我们定义了四个基本的成员函数来执行加减乘除操作,同时提供了默认参数来支持单数操作。构造函数负责初始化`num1`和`num2`为0,而析构函数不做特别的操作,通常在程序结束时自动调用。
阅读全文
相关推荐
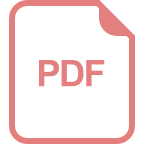
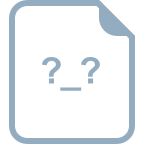
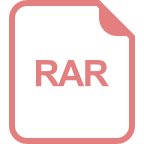
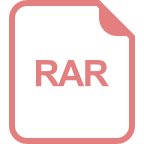
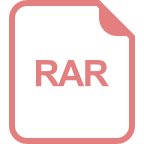
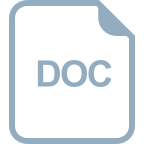
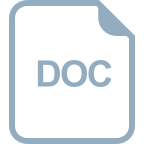


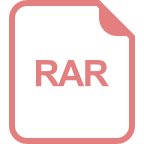
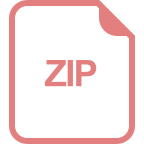
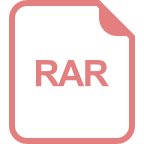
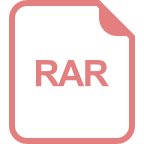
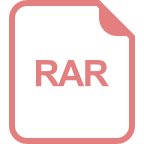
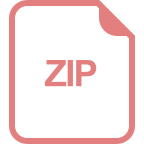
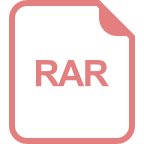
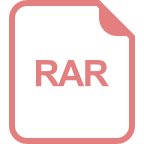
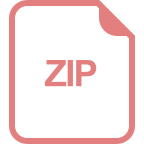