def sphericalToCartesianPointCloud(sphericalPointCloud): shape = sphericalPointCloud.shape cartestianPointCloud = sphericalPointCloud.copy() if (shape[1] < 3): print('Error: Failed to convert spherical point cloud to cartesian due to numpy array with too few dimensions') return sphericalPointCloud # Compute X # Range * sin (azimuth) * cos (elevation) cartestianPointCloud[:,0] = sphericalPointCloud[:,0] * np.sin(sphericalPointCloud[:,1]) * np.cos(sphericalPointCloud[:,2]) # Compute Y # Range * cos (azimuth) * cos (elevation) cartestianPointCloud[:,1] = sphericalPointCloud[:,0] * np.cos(sphericalPointCloud[:,1]) * np.cos(sphericalPointCloud[:,2]) # Compute Z # Range * sin (elevation) cartestianPointCloud[:,2] = sphericalPointCloud[:,0] * np.sin(sphericalPointCloud[:,2]) return cartestianPointCloud翻译一下这段代码
时间: 2024-04-03 21:32:10 浏览: 110
这段代码是一个将球面坐标系点云转换为笛卡尔坐标系点云的函数。函数名为sphericalToCartesianPointCloud,它接受一个球面坐标系点云的numpy数组作为输入参数sphericalPointCloud。
函数首先检查输入数组的维度是否足够,如果不足,则返回原始球面坐标系点云。
接下来,函数根据球面坐标系中的半径、方位角和仰角计算出笛卡尔坐标系中的x、y和z坐标,并将其存储在新的numpy数组cartesianPointCloud中。具体计算公式如下:
- x坐标:半径 * sin(方位角) * cos(仰角)
- y坐标:半径 * cos(方位角) * cos(仰角)
- z坐标:半径 * sin(仰角)
最后,函数返回新的笛卡尔坐标系点云numpy数组cartesianPointCloud。
相关问题
def __getitem__(self, index): while(self.index+self.TreePoint>self.datalen): filename = self.dataNames[self.fileIndx] # print(filename) if self.dataBuffer: a = [self.dataBuffer[0][self.index:].copy()] else: a=[] cell,mat = self.loader(filename) for i in range(cell.shape[1]): data = np.transpose(mat[cell[0,i]]) #shape[ptNum,Kparent, Seq[1],Level[1],Octant[1],Pos[3] ] e.g 123456*7*6 data[:,:,0] = data[:,:,0] - 1 a.append(data[:,-levelNumK:,:])# only take levelNumK level feats self.dataBuffer = [] self.dataBuffer.append(np.vstack(tuple(a))) self.datalen = self.dataBuffer[0].shape[0] self.fileIndx+=1 # shuffle step = 1, will load continuous mat self.index = 0 if(self.fileIndx>=self.fileLen): self.fileIndx=index%self.fileLen # try read img = [] img.append(self.dataBuffer[0][self.index:self.index+self.TreePoint]) self.index+=self.TreePoint if self.transform is not None: img = self.transform(img) return img
这段代码定义了 `DataFolder` 类的 `__getitem__` 方法,用于根据给定的索引 `index` 获取数据集中的某个样本。
方法的实现如下:
1. 首先,通过 while 循环判断当前索引加上树结构节点数量是否超过当前数据集的长度 `self.datalen`。如果超过,则表示当前文件中的数据已经被完全使用,需要加载下一个文件。
2. 在 while 循环中,首先获取下一个文件名 `filename`。
3. 如果当前数据缓冲区 `self.dataBuffer` 不为空,则将其第一个元素中从当前索引开始到末尾的部分复制给列表 `a`。否则,将 `a` 初始化为空列表。
4. 调用加载器 `self.loader(filename)` 并将返回的 `cell` 和 `mat` 赋值给对应的变量。
5. 使用循环遍历 `cell` 的第二个维度,即 `cell.shape[1]`,并在每次迭代中获取 `mat[cell[0, i]]` 的转置结果,并将其减去1。然后,将该结果的最后一维(Pos 维度)中的最后 `levelNumK` 个元素切片出来,并将其添加到列表 `a` 中。
6. 清空数据缓冲区,并将列表 `a` 中的所有元素按垂直方向堆叠起来,形成一个新的数据缓冲区,并将其赋值给 `self.dataBuffer`。
7. 更新数据集的长度 `self.datalen` 为新的数据缓冲区的长度。
8. 增加文件索引 `self.fileIndx` 的值,以加载下一个文件。
9. 将索引 `self.index` 重置为0。
10. 如果文件索引 `self.fileIndx` 超过了文件数量 `self.fileLen`,则将文件索引设置为 `index` 对文件数量取模的结果,以确保循环使用文件。
11. 尝试读取数据缓冲区中从 `self.index` 开始到 `self.index+self.TreePoint` 结束的部分,并将其添加到列表 `img` 中。
12. 将索引 `self.index` 增加树结构节点数量,以便下一次获取样本时可以继续读取数据缓冲区中的下一个部分。
13. 如果定义了数据转换操作 `self.transform`,则将列表 `img` 应用该转换操作。
14. 最后,返回转换后的列表 `img`。
这段代码通过循环加载数据并维护一个数据缓冲区,以实现按需加载数据,同时提供了一些额外的功能来处理数据集的边界情况。
rom osgeo import gdal import copy import numpy as np from PIL import Image from tqdm import tqdm # read image def readTif(fileName): dataset = gdal.Open(fileName) if dataset == None: print(fileName + "文件无法打开") width = dataset.RasterXSize # 栅格矩阵的列数 height = dataset.RasterYSize # 栅格矩阵的行数 data = dataset.ReadAsArray(0, 0, width, height) return data, dataset # 保存tif文件函数 def writeTiff(im_data, im_geotrans, im_proj, path): if 'int8' in im_data.dtype.name: datatype = gdal.GDT_Byte elif 'int16' in im_data.dtype.name: datatype = gdal.GDT_UInt16 else: datatype = gdal.GDT_Float32 if len(im_data.shape) == 3: im_bands, im_height, im_width = im_data.shape elif len(im_data.shape) == 2: im_data = np.array([im_data]) im_bands, im_height, im_width = im_data.shape # 创建文件 driver = gdal.GetDriverByName("GTiff") dataset = driver.Create(path, int(im_width), int(im_height), int(im_bands), datatype) if (dataset != None): dataset.SetGeoTransform(im_geotrans) # 写入仿射变换参数 dataset.SetProjection(im_proj) # 写入投影 for i in range(im_bands): dataset.GetRasterBand(i + 1).WriteArray(im_data[i]) del dataset
你可以使用 Lua 的 io 库来读取和写入文件。以下是一个简单的 Lua 脚本,可以将 lvmvm 文件转换为 txt 文件:
```lua
-- 打开 lvmvm 文件
local f = io.open("input.lvmvm", "rb")
-- 读取文件内容
local content = f:read("*all")
-- 关闭文件
f:close()
-- 将 lvmvm 文件内容写入 txt 文件
local f = io.open("output.txt", "w")
f:write(content)
f:close()
```
请将 "input.lvmvm" 替换为你要转换的 lvmvm 文件的路径,将 "output.txt" 替换为你要输出的 txt 文件的路径。
阅读全文
相关推荐
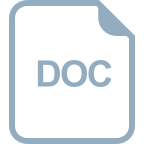
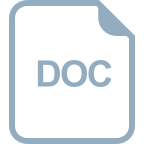
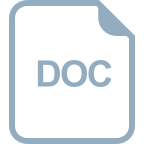














