ros中使用deepsort
时间: 2024-01-03 14:04:58 浏览: 76
在ROS中使用DeepSORT,需要安装相关依赖包和配置环境:
1. 安装Python 3.x和pip包管理器;
2. 安装TensorFlow和OpenCV等相关依赖包;
3. 下载DeepSORT源代码并进行编译安装;
4. 在ROS应用程序中调用DeepSORT API进行目标跟踪。
以下是一个可能的ROS节点示例代码,可以使用DeepSORT跟踪摄像头中的目标:
```python
#!/usr/bin/env python
import rospy
import cv2
import numpy as np
from sensor_msgs.msg import Image
from cv_bridge import CvBridge, CvBridgeError
from deep_sort_tracker import DeepSortTracker
class ObjectTracker:
def __init__(self):
self.bridge = CvBridge()
self.deepsort = DeepSortTracker()
self.image_sub = rospy.Subscriber("/camera/image_raw", Image, self.image_callback)
self.image_pub = rospy.Publisher("/camera/image_tracked", Image, queue_size=10)
def image_callback(self, data):
try:
cv_image = self.bridge.imgmsg_to_cv2(data, "bgr8")
except CvBridgeError as e:
rospy.logerr(e)
boxes, classes, scores = self.deepsort.track(cv_image)
for i in range(len(boxes)):
box = boxes[i]
class_name = classes[i]
score = scores[i]
# Draw bounding box and label
cv2.rectangle(cv_image, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), 2)
cv2.putText(cv_image, f"{class_name} {score:.2f}", (box[0], box[1] - 10),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
try:
self.image_pub.publish(self.bridge.cv2_to_imgmsg(cv_image, "bgr8"))
except CvBridgeError as e:
rospy.logerr(e)
if __name__ == '__main__':
rospy.init_node('object_tracker', anonymous=True)
object_tracker = ObjectTracker()
try:
rospy.spin()
except KeyboardInterrupt:
print("Shutting down")
```
其中,DeepSortTracker是一个自定义的类,包含了DeepSORT的初始化和跟踪方法。在image_callback中调用DeepSortTracker.track方法进行目标跟踪,将跟踪结果发布到ROS话题中。
阅读全文
相关推荐
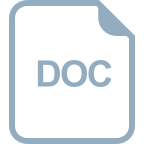
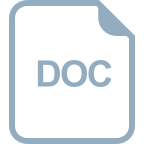
















