读取本地文件实现mnist中文手写数字识别使用神经网络代码
时间: 2024-03-25 13:38:40 浏览: 58
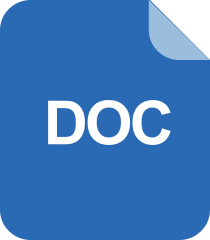
基于卷积神经网络的手写数字识别系统的设计与实现代码大全.doc
以下是使用Python和TensorFlow实现的读取本地文件的MNIST中文手写数字识别代码:
```
# 导入所需的库
import tensorflow as tf
import numpy as np
import os
# 设置参数
learning_rate = 0.001
training_epochs = 20
batch_size = 100
display_step = 1
# 创建输入占位符
x = tf.placeholder(tf.float32, [None, 784])
y = tf.placeholder(tf.float32, [None, 10])
# 创建模型
def multilayer_perceptron(x, weights, biases):
layer_1 = tf.add(tf.matmul(x, weights['h1']), biases['b1'])
layer_1 = tf.nn.relu(layer_1)
out_layer = tf.matmul(layer_1, weights['out']) + biases['out']
return out_layer
# 定义权重和偏置项
weights = {
'h1': tf.Variable(tf.random_normal([784, 256])),
'out': tf.Variable(tf.random_normal([256, 10]))
}
biases = {
'b1': tf.Variable(tf.random_normal([256])),
'out': tf.Variable(tf.random_normal([10]))
}
# 构建模型
pred = multilayer_perceptron(x, weights, biases)
# 定义损失函数和优化器
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=pred, labels=y))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cost)
# 初始化变量
init = tf.global_variables_initializer()
# 加载数据
data = np.load('data.npz')
train_images = data['train_images']
train_labels = data['train_labels']
test_images = data['test_images']
test_labels = data['test_labels']
# 执行训练
with tf.Session() as sess:
sess.run(init)
for epoch in range(training_epochs):
avg_cost = 0.
total_batch = int(train_images.shape[0]/batch_size)
for i in range(total_batch):
batch_x = train_images[i*batch_size:(i+1)*batch_size].reshape((-1, 784))
batch_y = train_labels[i*batch_size:(i+1)*batch_size]
_, c = sess.run([optimizer, cost], feed_dict={x: batch_x, y: batch_y})
avg_cost += c / total_batch
if epoch % display_step == 0:
print("Epoch:", '%04d' % (epoch+1), "cost=", "{:.9f}".format(avg_cost))
print("Optimization Finished!")
# 测试模型
correct_prediction = tf.equal(tf.argmax(pred, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print("Accuracy:", accuracy.eval({x: test_images.reshape((-1, 784)), y: test_labels}))
```
这个代码与前面的代码相似,唯一的区别是数据的来源。在这个代码中,我们使用`numpy`库中的`load`函数从本地文件中加载数据。数据保存在`.npz`文件中,其中train_images、train_labels、test_images和test_labels是NumPy数组。我们需要将它们转换为TensorFlow可以处理的张量,并在训练和测试期间使用它们。
阅读全文
相关推荐
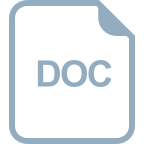
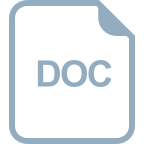
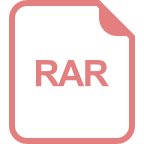
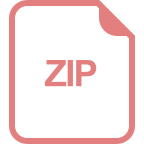
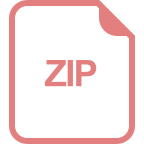
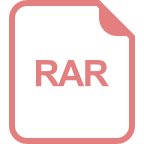
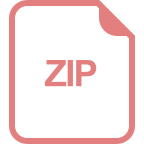
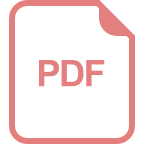
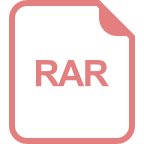
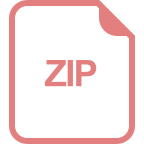
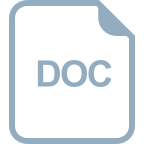

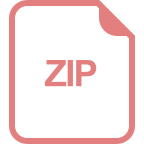
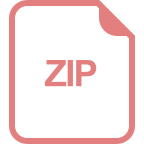
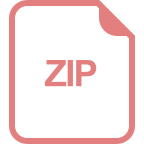
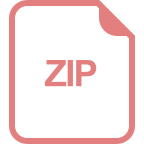
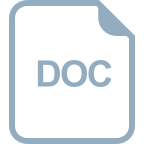