写个五子棋java代码
时间: 2023-08-31 08:41:03 浏览: 67
### 回答1:
好的,以下是一个简单的五子棋Java代码示例:
```
import java.util.Scanner;
public class FiveChess {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int[][] chessboard = new int[15][15];
boolean isBlack = true;
boolean isOver = false;
while (!isOver) {
int x, y;
System.out.print("请输入落子位置,以逗号分隔(如:3,5):");
String[] position = input.nextLine().split(",");
x = Integer.parseInt(position[0]) - 1;
y = Integer.parseInt(position[1]) - 1;
if (chessboard[x][y] != 0) {
System.out.println("该位置已有棋子,请重新输入!");
continue;
}
if (isBlack) {
chessboard[x][y] = 1; // 黑子
} else {
chessboard[x][y] = 2; // 白子
}
// 打印棋盘
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
System.out.print(chessboard[i][j] + " ");
}
System.out.println();
}
// 判断游戏是否结束
if (isWin(chessboard, x, y)) {
if (isBlack) {
System.out.println("黑方胜利!");
} else {
System.out.println("白方胜利!");
}
isOver = true;
}
isBlack = !isBlack;
}
}
// 判断游戏是否结束
public static boolean isWin(int[][] chessboard, int x, int y) {
int color = chessboard[x][y];
int count = 1;
// 判断横向是否五子连珠
for (int i = x - 1; i >= 0; i--) {
if (chessboard[i][y] == color) {
count++;
} else {
break;
}
}
for (int i = x + 1; i < 15; i++) {
if (chessboard[i][y] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断纵向是否五子连珠
count = 1;
for (int j = y - 1; j >= 0; j--) {
if (chessboard[x][j] == color) {
count++;
} else {
break;
}
}
for (int j = y + 1; j < 15; j++) {
if (chessboard[x][j] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断左上右下是否五子连珠
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--) {
if (chessboard[i][j] == color) {
count++;
} else {
break;
}
}
for (int
### 回答2:
五子棋是一种黑白棋类游戏,有很多种实现方式。下面是一个简单的五子棋Java代码实现:
```java
import java.util.Scanner;
public class FiveChess {
private static final int SIZE = 15;
private static final char BLANK = ' ';
private static final char BLACK = '●';
private static final char WHITE = '○';
private static char[][] board = new char[SIZE][SIZE];
public static void main(String[] args) {
initializeBoard();
boolean isBlack = true; // 当前落子颜色,默认为黑色
boolean isWin = false; // 是否获胜
while (!isWin) {
printBoard();
char currentPiece = isBlack ? BLACK : WHITE;
System.out.println((isBlack ? "黑" : "白") + "方,请输入落子的横纵坐标(以空格分隔,例如3 4):");
Scanner scanner = new Scanner(System.in);
int x = scanner.nextInt();
int y = scanner.nextInt();
if (!isValidMove(x, y)) {
System.out.println("该位置无法落子,请重新输入!");
continue;
}
placePiece(x, y, currentPiece);
isWin = checkWin(x, y, currentPiece);
isBlack = !isBlack;
}
System.out.println(((isBlack ? "白" : "黑") + "方胜利!"));
}
private static void initializeBoard() {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
board[i][j] = BLANK;
}
}
}
private static void printBoard() {
System.out.print(" ");
for (int i = 0; i < SIZE; i++) {
System.out.printf("%2d ", i + 1);
}
System.out.println();
for (int i = 0; i < SIZE; i++) {
System.out.printf("%2d ", i + 1);
for (int j = 0; j < SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
private static boolean isValidMove(int x, int y) {
return x >= 0 && x < SIZE && y >= 0 && y < SIZE && board[x][y] == BLANK;
}
private static void placePiece(int x, int y, char piece) {
board[x][y] = piece;
}
private static boolean checkWin(int x, int y, char piece) {
int count = 1; // 连子数
// 横向检查
for (int i = y - 1; i >= 0 && board[x][i] == piece; i--) {
count++;
}
for (int i = y + 1; i < SIZE && board[x][i] == piece; i++) {
count++;
}
if (count >= 5) {
return true;
}
// 纵向检查
count = 1;
for (int i = x - 1; i >= 0 && board[i][y] == piece; i--) {
count++;
}
for (int i = x + 1; i < SIZE && board[i][y] == piece; i++) {
count++;
}
if (count >= 5) {
return true;
}
// 正斜向检查
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == piece; i--, j--) {
count++;
}
for (int i = x + 1, j = y + 1; i < SIZE && j < SIZE && board[i][j] == piece; i++, j++) {
count++;
}
if (count >= 5) {
return true;
}
// 反斜向检查
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < SIZE && board[i][j] == piece; i--, j++) {
count++;
}
for (int i = x + 1, j = y - 1; i < SIZE && j >= 0 && board[i][j] == piece; i++, j--) {
count++;
}
return count >= 5;
}
}
```
这段代码实现了一个简单的五子棋游戏。游戏通过控制台进行交互,玩家依次输入落子的横坐标和纵坐标,然后将棋子落在对应的位置上。程序会判断每次落子后是否有一方胜利。游戏结束后会打印出胜利方。代码中的棋盘使用一个二维字符数组来表示,其中空格表示空白,黑色棋子使用"●"表示,白色棋子使用"○"表示。
### 回答3:
五子棋是一种非常受欢迎的棋类游戏,下面是一个简单的五子棋Java代码实现。
```java
import java.util.Scanner;
public class FiveInARow {
private static final int SIZE = 15; // 棋盘大小
private static final char EMPTY = ' '; // 空位
private static final char BLACK = '●'; // 黑子
private static final char WHITE = '○'; // 白子
private char[][] board; // 棋盘
private char currentPlayer; // 当前下棋玩家
public FiveInARow() {
board = new char[SIZE][SIZE];
currentPlayer = BLACK;
// 初始化棋盘
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
board[i][j] = EMPTY;
}
}
}
// 交换下棋玩家
private void switchPlayer() {
if (currentPlayer == BLACK) {
currentPlayer = WHITE;
} else {
currentPlayer = BLACK;
}
}
// 下棋
private void makeMove(int row, int col) {
if (isValidMove(row, col)) {
board[row][col] = currentPlayer;
switchPlayer();
} else {
System.out.println("无效的落子位置!");
}
}
// 判断落子位置是否合法
private boolean isValidMove(int row, int col) {
return row >= 0 && row < SIZE && col >= 0 && col < SIZE && board[row][col] == EMPTY;
}
// 判断游戏是否结束
private boolean isGameOver(int row, int col) {
return checkHorizontal(row, col) || checkVertical(row, col) || checkDiagonal1(row, col) || checkDiagonal2(row, col);
}
// 检查水平方向是否五子连珠
private boolean checkHorizontal(int row, int col) {
int count = 1;
// 向左查找
for (int i = col - 1; i >= 0 && board[row][i] == currentPlayer; i--) {
count++;
}
// 向右查找
for (int i = col + 1; i < SIZE && board[row][i] == currentPlayer; i++) {
count++;
}
return count >= 5;
}
// 检查垂直方向是否五子连珠
private boolean checkVertical(int row, int col) {
int count = 1;
// 向上查找
for (int i = row - 1; i >= 0 && board[i][col] == currentPlayer; i--) {
count++;
}
// 向下查找
for (int i = row + 1; i < SIZE && board[i][col] == currentPlayer; i++) {
count++;
}
return count >= 5;
}
// 检查正对角线方向是否五子连珠
private boolean checkDiagonal1(int row, int col) {
int count = 1;
// 向左上查找
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0 && board[i][j] == currentPlayer; i--, j--) {
count++;
}
// 向右下查找
for (int i = row + 1, j = col + 1; i < SIZE && j < SIZE && board[i][j] == currentPlayer; i++, j++) {
count++;
}
return count >= 5;
}
// 检查反对角线方向是否五子连珠
private boolean checkDiagonal2(int row, int col) {
int count = 1;
// 向左下查找
for (int i = row + 1, j = col - 1; i < SIZE && j >= 0 && board[i][j] == currentPlayer; i++, j--) {
count++;
}
// 向右上查找
for (int i = row - 1, j = col + 1; i >= 0 && j < SIZE && board[i][j] == currentPlayer; i--, j++) {
count++;
}
return count >= 5;
}
// 打印棋盘
private void printBoard() {
System.out.print(" ");
for (int i = 0; i < SIZE; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < SIZE; i++) {
System.out.print(i + " ");
for (int j = 0; j < SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
FiveInARow game = new FiveInARow();
Scanner scanner = new Scanner(System.in);
int row, col;
while (true) {
game.printBoard();
System.out.println(game.currentPlayer + " 下棋,请输入坐标(行 列):");
row = scanner.nextInt();
col = scanner.nextInt();
game.makeMove(row, col);
if (game.isGameOver(row, col)) {
System.out.println(game.currentPlayer + " 胜利!");
break;
}
}
scanner.close();
}
}
```
这个代码实现了一个基本的五子棋游戏,使用字符数组作为棋盘,通过控制台输入坐标控制下棋的位置。游戏会判断是否有五子连珠从而判断游戏胜负。以上是一个简单的实现,可根据需求进一步完善。
相关推荐
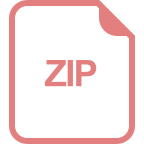
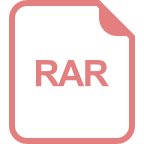














