帮我写一段Android代码,实现如下功能:检测userdata分区的大小,如果小于某个值,则提示用户系统空间不足无法写入
时间: 2024-12-19 16:28:45 浏览: 10
在Android中,你可以使用`StorageManager` API 来获取设备的存储信息,包括内部存储空间。以下是一个简单的示例代码片段,它检查userdata分区的可用空间,并在空间不足时显示一条通知:
```java
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.content.Context;
import android.content.pm.PackageManager;
import android.os.Environment;
import android.util.Log;
public class StorageSpaceChecker {
private static final String TAG = "StorageChecker";
private static final int NOTIFICATION_ID = 1001;
private static final int MIN_AVAILABLE_SPACE_GB = 5; // 设置最小可用空间为5GB
private Context context;
private NotificationManager notificationManager;
public StorageSpaceChecker(Context context) {
this.context = context;
notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
}
public void checkAndNotify() {
try {
long totalSize = Environment.getDataDirectory().getAvailableSpace();
float availableGb = totalSize / (1024 * 1024 * 1024); // 转换为GB
if (availableGb < MIN_AVAILABLE_SPACE_GB) {
Log.d(TAG, "Not enough storage space for userdata partition");
createNotification("存储警告", "您的内部存储空间不足 (" + (availableGb) + "GB),无法继续写入数据.");
} else {
Log.d(TAG, "Enough storage space for userdata partition");
}
} catch (Exception e) {
Log.e(TAG, "Error checking storage space", e);
}
}
private void createNotification(String title, String message) {
NotificationChannel channel = new NotificationChannel(NOTIFICATION_ID, "Storage Channel", NotificationManager.IMPORTANCE_DEFAULT);
channel.setDescription("存储空间警报");
context.getApplicationContext().registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
notificationManager.cancel(NOTIFICATION_ID);
}
}, new IntentFilter(Intent.ACTION_USER_PRESENT));
notificationManager.createNotificationChannel(channel);
Notification notification = new NotificationCompat.Builder(context, NOTIFICATION_ID)
.setSmallIcon(R.drawable.ic_storage_full)
.setContentTitle(title)
.setContentText(message)
.build();
notification.flags |= Notification.FLAG_AUTO_CANCEL;
notificationManager.notify(NOTIFICATION_ID, notification);
}
}
// 使用该类时,在需要的地方创建并调用
public static void main(String[] args) {
Context appContext = YourApplication.getAppContext(); // 替换为实际的上下文
StorageSpaceChecker checker = new StorageSpaceChecker(appContext);
checker.checkAndNotify();
}
```
这个代码会周期性地检查,并在满足条件时显示通知。注意,你需要在应用运行时请求访问存储权限。
阅读全文
相关推荐
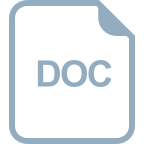
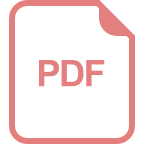
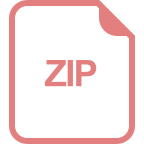















