请你扮演一个精通opencv的高手,使用opencv写一段伽马变换的代码,使用visual studio。要求拖动进度条实现伽马值的选取
时间: 2024-05-15 20:16:34 浏览: 10
以下是使用OpenCV和Visual Studio实现伽马变换并使用进度条选择伽马值的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <opencv2/highgui.hpp>
using namespace cv;
int gammaValue = 100; // 伽马值,初始化为100
void onTrackbar(int value, void* userData) {
gammaValue = value;
}
int main(int argc, char** argv) {
// 加载图像
Mat image = imread("path/to/image.jpg", IMREAD_COLOR);
if (image.empty()) {
printf("Could not open or find the image\n");
return -1;
}
// 创建窗口
namedWindow("Gamma Transformation", WINDOW_NORMAL);
resizeWindow("Gamma Transformation", 800, 600);
// 创建进度条
createTrackbar("Gamma Value", "Gamma Transformation", &gammaValue, 500, onTrackbar);
// 循环
while (true) {
// 计算伽马值
double gamma = gammaValue / 100.0;
// 进行伽马变换
Mat gammaImage;
cv::pow(image / 255.0, gamma, gammaImage);
gammaImage *= 255;
// 显示图像
imshow("Gamma Transformation", gammaImage);
// 检测按键
int key = waitKey(30);
if (key == 27) { // ESC
break;
}
}
return 0;
}
```
在代码中,我们首先加载了一个图像,然后创建了一个窗口和一个进度条。进度条的最小值为0,最大值为500,初始值为100。我们还定义了一个回调函数`onTrackbar`,它将在进度条值发生变化时被调用,将伽马值更新为滑块的当前值。
在循环中,我们首先计算伽马值(将滑块值除以100),然后使用`cv::pow`函数对图像进行伽马变换。最后,我们将变换后的图像显示在窗口中,并等待用户按下ESC键退出程序。
要运行此示例,您需要将图像路径替换为实际图像的路径。您还需要在项目属性中添加OpenCV的头文件和库路径,并将OpenCV库添加到链接器输入中。
相关推荐
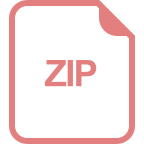
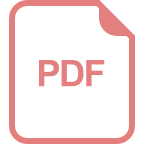
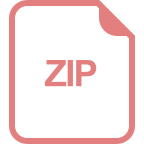












