std::chrono
时间: 2023-09-29 12:05:23 浏览: 45
`std::chrono`是C++11引入的时间库,用于对时间进行精确测量和处理。它提供了一个标准的、跨平台的时间库,可以方便地进行时间点的计算、时钟的处理、时间间隔的操作等。它与C语言中的time.h库不同,它提供了更高的精度和更多的功能。
`std::chrono`包含了三个主要的组件:
1. `std::chrono::duration`:表示一个时间间隔,可以是秒、毫秒、微秒、纳秒等等。
2. `std::chrono::time_point`:表示一个时间点,可以是系统时钟、高分辨率时钟等等。
3. `std::chrono::clock`:表示一个时钟,用于获取当前时间点。
使用`std::chrono`可以方便地进行时间的计算和处理,可以避免一些常见的时间处理问题,如时区、夏令时等等。
相关问题
std::chrono::
std::chrono是C++标准库中的一个时间库,它定义了许多常用的时间单位,包括hours、minutes、seconds、milliseconds、microseconds和nanoseconds。这些时间单位可以用来表示不同的时间间隔。
在std::chrono中,我们可以使用各种预定义的duration类来表示时间。这些类型是模板类std::chrono::duration的特化版本,它们接受一个表示时长的整数作为构造参数。
另外,std::chrono还提供了steady_clock类来表示稳定的时钟,我们可以使用它来测量时间间隔。通过调用steady_clock的now()函数可以获取当前时间点,然后可以使用time_point和duration来计算时间间隔。
下面是一个示例代码,展示了如何使用std::chrono来计算时间间隔:
```cpp
#include <iostream>
#include <chrono>
int main() {
std::chrono::steady_clock::time_point tp1 = std::chrono::steady_clock::now();
std::cout << "1970.1.1-----now:" << tp1.time_since_epoch().count() << std::endl;
int i = 100;
while(i) i--;
std::chrono::steady_clock::time_point tp2 = std::chrono::steady_clock::now();
std::chrono::duration<double> dur = tp2 - tp1;
std::cout << "time duration=" << dur.count() << std::endl;
return 0;
}
```
以上代码中,我们通过steady_clock的now()函数获取了两个时间点tp1和tp2,并使用duration计算了它们之间的时间间隔dur。
std::chrono::毫秒
std::chrono::毫秒是C++标准库中的一个时间单位,用于表示精确到毫秒的时间间隔或时间点。它是C++11引入的std::chrono库的一部分。
std::chrono::毫秒是一个持续时间类型,用于表示从一个时间点到另一个时间点的时间间隔。它可以用于测量代码执行时间、计时等需要精确度到毫秒级别的场景。
使用std::chrono::毫秒需要包含<chrono>头文件,并使用std::chrono::milliseconds命名空间。
以下是std::chrono::毫秒的一些常见用法:
1. 创建std::chrono::毫秒对象:
std::chrono::milliseconds duration(1000); // 表示1000毫秒
2. 获取std::chrono::毫秒对象的值:
auto value = duration.count(); // 获取持续时间的毫秒数,返回一个长整型
3. 进行时间间隔的计算:
std::chrono::milliseconds duration1(2000);
std::chrono::milliseconds duration2(1000);
auto diff = duration1 - duration2; // 计算两个持续时间的差值
4. 进行时间点的计算:
std::chrono::system_clock::time_point start = std::chrono::system_clock::now();
// 执行一些操作
std::chrono::system_clock::time_point end = std::chrono::system_clock::now();
std::chrono::milliseconds duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
auto duration_value = duration.count(); // 获取两个时间点之间的毫秒数
相关推荐
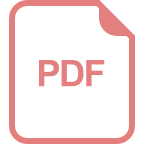
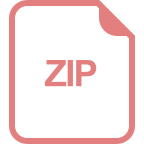
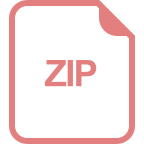
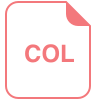











