python调库使用遗传算法
时间: 2023-12-22 22:27:38 浏览: 78
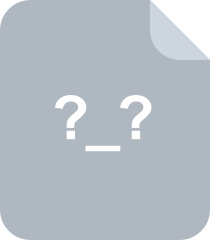
遗传算法python
根据提供的引用内容,可以看出Python中使用遗传算法需要调用numpy、random和matplotlib.pyplot等库。下面是一个简单的遗传算法示例,用于解决一个整数规划问题:
```python
import numpy as np
import random
import matplotlib.pyplot as plt
# 定义目标函数
def fitness_func(x):
return x[0] + x[1] - 2 * x[2]
# 定义种群初始化函数
def init_population(pop_size, chrom_length):
population = np.zeros((pop_size, chrom_length))
for i in range(pop_size):
population[i] = np.random.randint(0,2, chrom_length)
return population
# 定义选择函数
def selection(population, fitness):
fitness_sum = np.sum(fitness)
probability = fitness / fitness_sum
cum_probability = np.cumsum(probability)
new_population = np.zeros(population.shape)
for i in range(population.shape[0]):
random_num = random.random()
for j in range(population.shape[0]):
if random_num <= cum_probability[j]:
new_population[i] = population[j]
break
return new_population
# 定义交叉函数
def crossover(population, pc):
new_population = np.zeros(population.shape)
for i in range(0, population.shape[0], 2):
if random.random() < pc:
crossover_point = random.randint(1, population.shape[1] - 1)
new_population[i] = np.concatenate((population[i][:crossover_point], population[i+1][crossover_point:]))
new_population[i+1] = np.concatenate((population[i+1][:crossover_point], population[i][crossover_point:]))
else:
new_population[i] = population[i]
new_population[i+1] = population[i+1]
return new_population
# 定义变异函数
def mutation(population, pm):
for i in range(population.shape[0]):
if random.random() < pm:
mutation_point = random.randint(0, population.shape[1] - 1)
population[i][mutation_point] = 1 - population[i][mutation_point]
return population
# 定义遗传算法主函数
def genetic_algorithm(pop_size, chrom_length, pc, pm, max_iter):
population = init_population(pop_size, chrom_length)
fitness = np.zeros(pop_size)
best_fitness = np.zeros(max_iter)
best_chromosome = np.zeros((max_iter, chrom_length))
for i in range(max_iter):
for j in range(pop_size):
fitness[j] = fitness_func(population[j])
best_fitness[i] = np.max(fitness)
best_chromosome[i] = population[np.argmax(fitness)]
population = selection(population, fitness)
population = crossover(population, pc)
population = mutation(population, pm)
return best_fitness, best_chromosome
# 测试遗传算法
pop_size = 50
chrom_length = 3
pc = 0.8
pm = 0.01
max_iter = 100
best_fitness, best_chromosome = genetic_algorithm(pop_size, chrom_length, pc, pm, max_iter)
# 输出结果
print("最优解:", best_chromosome[-1])
print("最优值:", best_fitness[-1])
# 绘制适应度曲线
plt.plot(best_fitness)
plt.xlabel("迭代次数")
plt.ylabel("适应度")
plt.show()
```
阅读全文
相关推荐
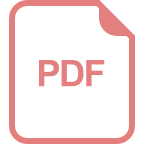









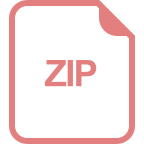





