pytesseract+selenium
时间: 2024-12-25 21:14:12 浏览: 5
Pytesseract 和 Selenium 是两个常用的 Python 库,它们各自解决的问题领域不同。
Pytesseract 是一个 OCR (Optical Character Recognition) 工具库,用于从图像中识别文本,它通常配合 Tesseract OCR 系统一起使用,Tesseract 是一个开源的文本识别引擎。通过 Pytesseract,你可以将截图、PDF 文件等图片内容转换为可编辑的文本数据,方便处理文档信息抓取和自动化。
Selenium 是一款广泛用于 Web 自动化的工具,主要用于模拟用户在浏览器上与网页交互的行为,如点击链接、填写表单、滚动页面等。它支持多种浏览器,比如 Chrome、Firefox 等,并能控制浏览器的行为,执行 JavaScript,获取和修改网页元素的内容。
当你结合使用 Pytesseract 和 Selenium 的时候,可能会有这样的场景:例如,在爬虫项目中,你首先使用 Selenium 执行浏览某个网站并截取含有重要信息的网页区域;然后,利用 Pytesseract 对这些截图进行OCR识别,提取出需要的数据,比如表格中的文字、网页元素的文字内容等。
相关问题
python + selenium 滑块自动验证
Python配合Selenium做滑块验证码自动验证的基本流程是这样的:
1. **安装依赖**:
首先,确保已经安装了`selenium`库,可以通过命令行执行 `pip install selenium` 完成安装。此外,还需要相应的浏览器驱动,例如Chromedriver或FirefoxDriver,根据你的浏览器类型选择合适的。
2. **启动浏览器**:
在Python脚本中初始化Selenium,创建一个`webdriver.Chrome()`或`webdriver.Firefox()`实例。为了不显示实际的浏览器窗口,可以设置`options`参数,如 `options.headless=True`。
```python
from selenium import webdriver
# 后续加上对应浏览器的选项
options = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
driver = webdriver.Chrome(options=options)
```
3. **导航到登录页面**:
使用`get`方法加载目标登录页面URL。
```python
login_url = "https://your-website.com/login"
driver.get(login_url)
```
4. **找到滑块元素**:
使用`find_element_by_*`方法定位滑块元素,如`find_element_by_id('captcha')`或`find_element_by_xpath('//img[contains(@class, "captcha")]')`等。这里假设滑块是一个图像元素。
```python
captcha_elem = driver.find_element_by_id('captcha')
```
5. **截取并处理滑块图片**:
用`screenshot_as_png`方法截图滑块,然后使用OpenCV或PIL库对图片进行预处理,包括灰度化、二值化等操作,以便后续的字符识别。
```python
import cv2
import numpy as np
# 获取滑块图片
captcha_image = captcha_elem.screenshot_as_png
# 读取图片
img = np.array(Image.open(BytesIO(captcha_image)))
# 对图片进行预处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
```
6. **字符识别**:
可能需要借助OCR(Optical Character Recognition)工具,如`tesseract`,将预处理后的图像转为文本。注意这步需要正确配置`tesseract`。
```python
from pytesseract import image_to_string
text = image_to_string(binary, lang='eng', config='--psm 11') # psm 11表示去除干扰线
```
7. **模拟滑动验证**:
根据识别出的文字,计算滑动条的位置并模拟鼠标移动。由于这是一个抽象的概念,具体实现取决于滑块验证码的具体形式。
8. **提交表单**:
输入滑块验证码之后,找到提交按钮或者其他验证通过的信号元素,如点击事件。
```python
captcha_input_field = driver.find_element_by_id('captcha_input') # 假设有一个input元素接受验证码
captcha_input_field.send_keys(text)
submit_button = driver.find_element_by_css_selector('#submit-button')
submit_button.click()
```
9. **等待验证完成**:
验证完成后,可能需要等待一段时间让滑块验证完成,例如使用`time.sleep()`。
10. **检查登录状态**:
检查登录后的行为或页面内容确认是否登录成功。
11. **关闭浏览器**:
最后,别忘了关闭浏览器会话。
```python
driver.quit()
```
请注意,滑块验证码的实现往往非常复杂,尤其当涉及到动态加载或实时更新时,上述过程可能不够准确。实际项目中可能需要更精细的处理和错误处理。另外,遵循网站的robots.txt规则以及尊重其反爬虫策略是非常重要的。
如何用python + selenium 来自动识别文字点选式验证码
### 回答1:
使用 Python + Selenium 自动识别文字点选式验证码的方法如下:
1. 安装 Selenium 库和对应的浏览器驱动;
2. 使用 Selenium 打开网页并获取验证码图片;
3. 使用 OCR 技术识别图片中的文字;
4. 使用 Selenium 点击图片中对应文字的位置。
代码示例:
```
from selenium import webdriver
from selenium.webdriver.common.by import By
# 启动浏览器
driver = webdriver.Chrome()
# 打开页面
driver.get("https://www.example.com/verify")
# 获取验证码图片并识别文字
code = recognize_verify_code(driver.find_element(By.CSS_SELECTOR, "#verify-code").screenshot_as_png)
# 点击图片中对应文字的位置
driver.find_element(By.CSS_SELECTOR, f"#verify-code .verify-item[title='{code}']").click()
```
其中 `recognize_verify_code` 函数需要自行实现,可以使用第三方 OCR 库,如 Tesseract。
### 回答2:
要使用Python和Selenium来自动识别文字点选式验证码,可以按照以下步骤进行:
1. 安装必要的软件和库:
- 安装Python:从Python官方网站下载并安装Python。
- 安装Selenium:使用pip命令安装Selenium库,可以使用以下命令:`pip install selenium`。
- 安装webdriver:下载并安装适合您浏览器版本的webdriver,可以使用chromedriver或geckodriver。
2. 导入所需的库和模块:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.by import By
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
```
3. 启动浏览器驱动程序:
```python
driver = webdriver.Chrome() # 如果使用Chrome浏览器
```
4. 打开目标网页:
```python
driver.get("目标网页的URL")
```
5. 定位验证码元素和识别区域:
```python
captcha_element = driver.find_element(By.XPATH, "验证码元素的XPath")
captcha_area = driver.find_element(By.XPATH, "识别区域的XPath")
```
6. 截取验证码图片:
```python
captcha_image = captcha_area.screenshot_as_png
```
7. 使用第三方验证码识别库处理验证码图片:
```python
# 这里假设使用了Tesseract OCR库来识别验证码
from PIL import Image
import pytesseract
captcha_text = pytesseract.image_to_string(Image.open(captcha_image))
```
8. 识别出的验证码文本作为答案,执行点击动作:
```python
actions = ActionChains(driver)
actions.move_to_element(captcha_element).click().send_keys(captcha_text).perform()
```
9. 提交验证码:
```python
submit_button = driver.find_element(By.XPATH, "提交按钮的XPath")
submit_button.click()
```
10. 等待页面加载完成,进行后续操作。
以上是一个基本的框架。具体步骤会根据网页的具体设计和验证码类型而有所不同。自动识别文字点选式验证码是一项复杂的任务,需要不断调试和优化识别算法。
阅读全文
相关推荐
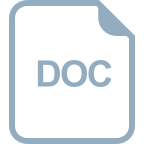
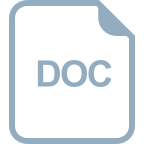
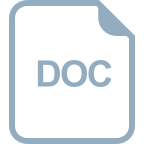
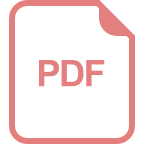
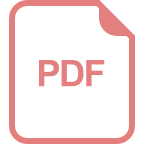
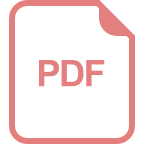
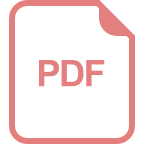
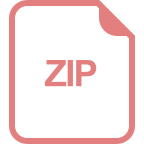








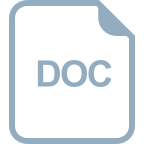