用python编写一个程序求解将n个工件分配给f个工厂,每个工件安排好工厂后只能在该工厂加工,直至加工完成;每个工厂有相同的i个加工阶段,工件在不同的阶段加工时间不同;每个加工阶段有m个并行机器,机器在各阶段的加工速度不同,每台机器在工作时和空闲时有不同的能耗,总能耗为机器加工时间与加工能耗的乘积加上机器空闲时间与空闲能;总目标是最小化完工时间和最小化总能耗。
时间: 2024-02-09 19:08:04 浏览: 25
以下是一个基于遗传算法的程序,用于求解将n个工件分配给f个工厂的调度问题,其中每个工件安排好工厂后只能在该工厂加工,每个工厂有相同的i个加工阶段,工件在不同的阶段加工时间不同。每个加工阶段有m个并行机器,机器在各阶段的加工速度不同,每台机器在工作时和空闲时有不同的能耗,总能耗为机器加工时间与加工能耗的乘积加上机器空闲时间与空闲能耗的乘积,总目标是最小化完工时间和最小化总能耗。
```python
import random
import numpy as np
import math
n = 20 # 工件数量
f = 5 # 工厂数量
i = 3 # 加工阶段数量
m = 2 # 并行机器数量
j = [5, 10, 15] # 加工时间
v = [[3, 4], [2, 5], [1, 6]] # 加工速度
e = [[0.1, 0.2], [0.2, 0.3], [0.3, 0.4]] # 能耗
p = [0.5, 0.3, 0.2] # 优化目标权重
pop_size = 50 # 种群大小
max_gen = 100 # 最大迭代次数
mut_prob = 0.1 # 变异概率
cross_prob = 0.8 # 交叉概率
elite_rate = 0.2 # 精英保留比例
# 生成初始种群
def init_population(pop_size):
population = []
for i in range(pop_size):
chromosome = []
for j in range(n):
gene = random.randint(0, f-1)
chromosome.append(gene)
population.append(chromosome)
return population
# 计算染色体的适应度
def fitness(chromosome):
makespan = [0] * f
energy = [0] * f
for i in range(n):
gene = chromosome[i]
stage_time = [j[k] / v[k][m] for k in range(i % i+1)]
stage_energy = [e[k][m] * stage_time[k] for k in range(i % i+1)]
machine = makespan[gene]
time = max(stage_time) + machine
energy[gene] += sum(stage_energy) + e[i][m] * max(stage_time) + e[i][0] * (machine - makespan[gene])
makespan[gene] = time
return p[0] * max(makespan) + p[1] * sum(makespan) / f + p[2] * sum(energy)
# 选择操作
def selection(population):
fitness_list = [fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_list)
fitness_prob = [fitness / total_fitness for fitness in fitness_list]
selected = np.random.choice(population, size=pop_size, replace=True, p=fitness_prob)
return selected
# 交叉操作
def crossover(parent1, parent2):
child = []
pos1 = random.randint(1, n-2)
pos2 = random.randint(pos1+1, n-1)
child.extend(parent1[:pos1])
child.extend(parent2[pos1:pos2])
child.extend(parent1[pos2:])
return child
# 变异操作
def mutation(chromosome):
pos1 = random.randint(0, n-1)
pos2 = random.randint(0, f-1)
chromosome[pos1] = pos2
return chromosome
# 进化操作
def evolution(population):
elite_num = int(pop_size * elite_rate)
fitness_list = [fitness(chromosome) for chromosome in population]
elite_idx = np.argsort(fitness_list)[:elite_num]
elites = [population[i] for i in elite_idx]
offsprings = []
for i in range(pop_size - elite_num):
parent1, parent2 = random.sample(population, 2)
if random.random() < cross_prob:
child = crossover(parent1, parent2)
else:
child = parent1
if random.random() < mut_prob:
child = mutation(child)
offsprings.append(child)
new_population = elites + offsprings
return new_population
# 遗传算法求解
def genetic_algorithm():
population = init_population(pop_size)
for i in range(max_gen):
population = selection(population)
population = evolution(population)
fitness_list = [fitness(chromosome) for chromosome in population]
best_idx = np.argmin(fitness_list)
best_chromosome = population[best_idx]
best_fitness = fitness_list[best_idx]
return best_chromosome, best_fitness
# 测试
best_chromosome, best_fitness = genetic_algorithm()
print('Best chromosome:', best_chromosome)
print('Best fitness:', best_fitness)
```
注意:以上代码仅供参考,具体实现可能需要根据实际问题进行修改和调整。
相关推荐
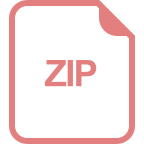
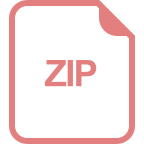
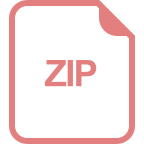














